使用libjpeg 对8bit图像进行压缩
时间: 2024-03-09 19:51:44 浏览: 63
可以使用libjpeg库对8bit图像进行压缩,具体步骤如下:
1. 引入libjpeg库的头文件:
```c
#include <jpeglib.h>
```
2. 定义需要压缩的图像数据和相关参数:
```c
JSAMPLE *image_buffer; // 图像数据
int image_width; // 图像宽度
int image_height; // 图像高度
int image_channels; // 图像通道数
```
3. 创建jpeg压缩对象:
```c
struct jpeg_compress_struct cinfo; // 压缩对象
struct jpeg_error_mgr jerr; // 错误处理对象
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_compress(&cinfo);
```
4. 设置压缩参数:
```c
cinfo.image_width = image_width;
cinfo.image_height = image_height;
cinfo.input_components = image_channels;
cinfo.in_color_space = JCS_RGB;
jpeg_set_defaults(&cinfo);
jpeg_set_quality(&cinfo, 80, TRUE);
```
5. 指定压缩输出目标:
```c
FILE *outfile;
outfile = fopen("output.jpg", "wb");
jpeg_stdio_dest(&cinfo, outfile);
```
6. 开始压缩:
```c
int row_stride = image_width * image_channels;
JSAMPROW row_pointer[1];
jpeg_start_compress(&cinfo, TRUE);
while (cinfo.next_scanline < cinfo.image_height) {
row_pointer[0] = &image_buffer[cinfo.next_scanline * row_stride];
jpeg_write_scanlines(&cinfo, row_pointer, 1);
}
jpeg_finish_compress(&cinfo);
fclose(outfile);
```
7. 释放压缩对象:
```c
jpeg_destroy_compress(&cinfo);
```
以上是使用libjpeg对8bit图像进行压缩的基本步骤。需要注意的是,压缩后的图像数据将保存在指定的输出文件中,可以在需要时读取并解压缩。
阅读全文
相关推荐
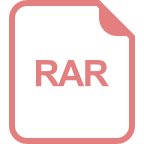
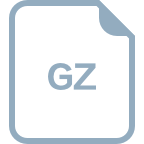




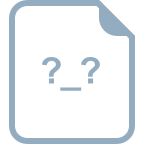
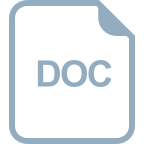
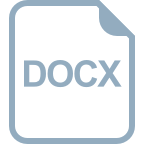
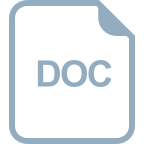
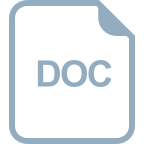
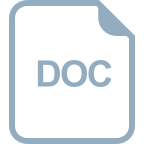




