java发送待确认提醒消息给前端
时间: 2023-11-25 15:54:30 浏览: 157
您可以使用Java中的WebSocket来实现向前端发送待确认提醒消息的功能。WebSocket是一种实时通信协议,它允许在客户端和服务器之间建立持久连接,可以实时地向客户端推送消息。
以下是一个简单的Java WebSocket实现示例:
```java
@ServerEndpoint("/websocket")
public class WebSocketServer {
private static Set<Session> sessions = Collections.synchronizedSet(new HashSet<>());
@OnOpen
public void onOpen(Session session) {
sessions.add(session);
}
@OnClose
public void onClose(Session session) {
sessions.remove(session);
}
@OnMessage
public void onMessage(String message, Session session) {
// 处理收到的消息
if (message.equals("confirm")) {
// 发送待确认提醒消息
String notification = "您有待确认的任务,请及时处理。";
for (Session s : sessions) {
s.getAsyncRemote().sendText(notification);
}
}
}
}
```
在上面的示例中,我们使用了@ServerEndpoint注解来指定WebSocket的端点地址。在onOpen方法中,我们将新的Session对象添加到sessions集合中。在onClose方法中,我们从sessions集合中删除已关闭的Session对象。在onMessage方法中,我们处理收到的消息,如果收到了"confirm"消息,就向所有客户端发送待确认提醒消息。
您可以在前端使用JavaScript来连接WebSocket,并处理接收到的消息:
```javascript
var websocket = new WebSocket("ws://localhost:8080/websocket");
websocket.onmessage = function(event) {
// 处理接收到的消息
var notification = event.data;
alert(notification);
};
function sendConfirmMessage() {
// 发送确认消息
websocket.send("confirm");
}
```
在上面的示例中,我们使用WebSocket的onmessage事件处理接收到的消息。当收到消息时,我们弹出一个警告框显示待确认提醒消息。在sendConfirmMessage函数中,我们向服务器发送确认消息。
阅读全文
相关推荐
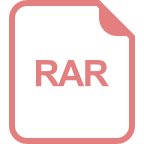
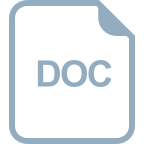
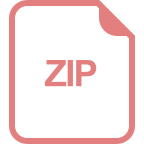
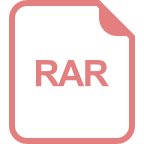
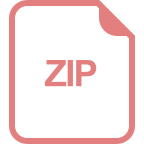
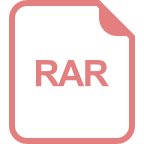
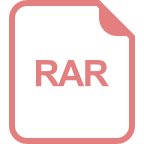
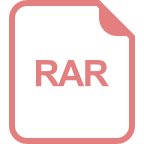
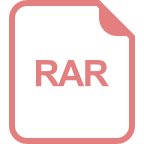
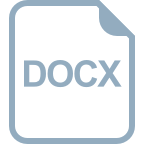
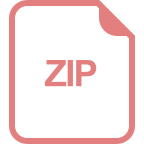
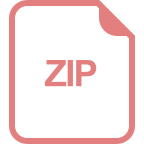
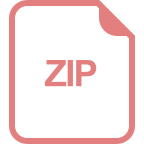
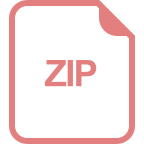
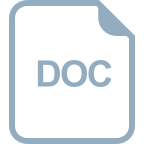
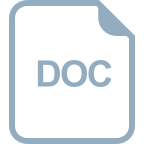
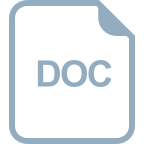
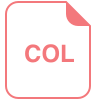