用java代码编写一个整体的程序,在IDEA里面实现,要求设计一个学生student类,包含学号、姓名、出生日期、性别等字段 从input.txt文件读取其中的学生信息,并存入学生集合list中 对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小) 将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt(内容为:1001 张三 1998-01-18 男 1002 李四 1999-04-28 男 1003 王五 1998-03-02 女 1004 赵六 1996-06-20 男 1005 孙七 1998-12-15 女 1006 周八 1997-09-09 女 1007 吴九 1998-10-18 男 1008 郑十 1999-05-13 女)
时间: 2024-02-18 07:03:20 浏览: 71
好的,以下是Java代码实现:
```java
import java.io.*;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.*;
public class Student implements Comparable<Student> {
private int id;
private String name;
private Date birthdate;
private String gender;
public Student(int id, String name, Date birthdate, String gender) {
this.id = id;
this.name = name;
this.birthdate = birthdate;
this.gender = gender;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public Date getBirthdate() {
return birthdate;
}
public String getGender() {
return gender;
}
@Override
public int compareTo(Student s) {
return this.birthdate.compareTo(s.getBirthdate());
}
@Override
public String toString() {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
return id + " " + name + " " + dateFormat.format(birthdate) + " " + gender;
}
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
try (Scanner scanner = new Scanner(new FileInputStream("input.txt"))) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] tokens = line.split(" ");
int id = Integer.parseInt(tokens[0]);
String name = tokens[1];
Date birthdate = new SimpleDateFormat("yyyy-MM-dd").parse(tokens[2]);
String gender = tokens[3];
students.add(new Student(id, name, birthdate, gender));
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (ParseException e) {
e.printStackTrace();
}
Collections.sort(students);
try (PrintWriter writer = new PrintWriter(new FileOutputStream("output.txt"))) {
for (Student student : students) {
writer.println(student.toString());
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
```
这个程序包括一个 `Student` 类,其中包含了学生的信息,以及一个 `main` 方法,用于读取文件、排序和输出文件。
在 `main` 方法中,我们首先创建了一个空的 `List<Student>` 对象,然后使用 `Scanner` 读取 `input.txt` 文件中的每一行,将每个学生信息解析为 `Student` 对象,并将其添加到列表中。然后,我们使用 `Collections.sort()` 方法对学生列表进行排序,该方法使用 `Student` 对象的 `compareTo()` 方法来比较学生的出生日期。最后,我们使用 `PrintWriter` 将排序后的学生列表输出到 `output.txt` 文件中。
请确保在运行程序之前,将包含学生信息的 `input.txt` 文件放在程序的同一目录下。
阅读全文
相关推荐
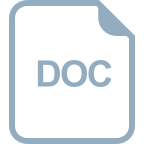
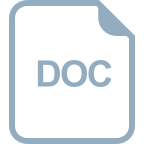
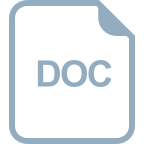




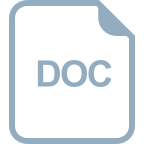







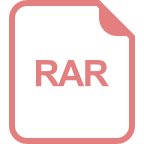
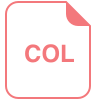

