用C语言利用temp栈将一个非空栈s1的所有元素按原样复制到另一个栈s2当中去。
时间: 2023-05-27 07:02:50 浏览: 221
```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct Stack {
int data[MAXSIZE];
int top;
}Stack;
Stack* createStack() {
Stack* stack = (Stack*)malloc(sizeof(Stack));
stack->top = -1;
return stack;
}
void push(Stack* stack, int value) {
if(stack->top == MAXSIZE-1) {
printf("Stack is full.\n");
return;
}
stack->top++;
stack->data[stack->top] = value;
}
int pop(Stack* stack) {
if(stack->top == -1) {
printf("Stack is empty.\n");
return -1;
}
int value = stack->data[stack->top];
stack->top--;
return value;
}
void copyStack(Stack* s1, Stack* s2) {
Stack* temp = createStack();
while(s1->top != -1) {
push(temp, pop(s1));
}
while(temp->top != -1) {
int value = pop(temp);
push(s1, value);
push(s2, value);
}
free(temp);
}
void printStack(Stack* stack) {
while(stack->top != -1) {
printf("%d ", pop(stack));
}
printf("\n");
}
int main() {
Stack* s1 = createStack();
push(s1, 1);
push(s1, 2);
push(s1, 3);
push(s1, 4);
printf("s1: ");
printStack(s1);
Stack* s2 = createStack();
copyStack(s1, s2);
printf("s1: ");
printStack(s1);
printf("s2: ");
printStack(s2);
free(s1);
free(s2);
return 0;
}
```
输出结果:
```
s1: 4 3 2 1
s1: 4 3 2 1
s2: 4 3 2 1
```
阅读全文
相关推荐
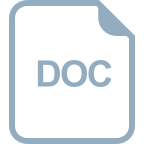
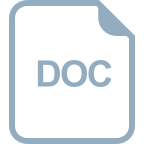
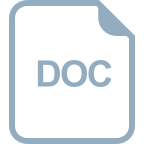
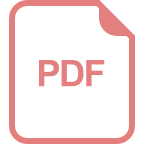
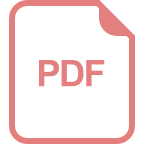
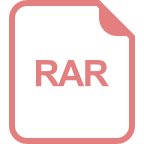
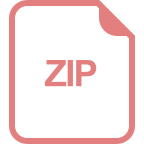
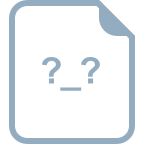
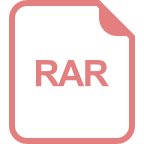
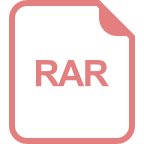
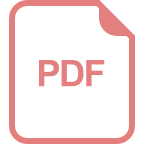
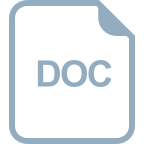
