优化代码void QQuickPrint::CalcCleanSprayInk(int nCleanSprayTime, int nCleanSprayStartTime, int nCleanSprayEndTime) { if (nCleanSprayTime <= 0) return; _CLEANSPRAY_INKINFO *stuCleanSprayInkInfo = new _CLEANSPRAY_INKINFO; stuCleanSprayInkInfo->nCostTime = nCleanSprayTime; stuCleanSprayInkInfo->nStartTime = static_cast<uint>(nCleanSprayStartTime); stuCleanSprayInkInfo->nEndTime = static_cast<uint>(nCleanSprayEndTime); stuCleanSprayInkInfo->nType = CLEANSPRAY_INK_CALCULATE; int nCntOfChannel = m_qPrintParam->GetCntOfChannel(); int nFrameSize = m_qPrintParam->GetFrameSize(); //喷头孔数 int nCleanDropSize = m_qPrintParam->GetCleanFireDropSize(); double dDropSizeCost = CLEANSPRAYDROPSIZE[nCleanDropSize];//清喷小点、中点、大点对应的耗墨量 int nCleanFireTimes = m_qPrintParam->GetCleanFireTimes(); int nCleanFireInterval = m_qPrintParam->GetCleanFireInterval(); int nCleanTotalTimes = (nCleanSprayTime / nCleanFireInterval) + 1;//清喷动作执行次数 = (清喷时间 / 清喷间隔) + 1,+1的原因是开启清喷时会立即执行1次清喷动作 //单通道清喷动作耗墨量 = 喷头孔数 * 清喷大小 * 单次清喷动作的清喷次数 * 清喷动作执行次数 double dColorCost = PL2ML(nFrameSize * dDropSizeCost * nCleanFireTimes * nCleanTotalTimes); memset(stuCleanSprayInkInfo->dInkCost, 0.00, sizeof(double) * MAXCOLORS); //获取各通道对应的颜色,计算各通道清喷耗墨量 for (int iC = 0; iC != nCntOfChannel; ++iC) { int nColorsCnt = m_qPrintParam->GetCntOfColors(); int nColorIndex = m_qPrintParam->GetRIPDataOfPiece(iC); if (PRN_CMYKOrRBLk == nColorsCnt) //8色模式,通道依次接RIP图的第7 6 1 3 0 2 5 4个位置 { //判断清喷通道接的RIP图位置对应哪个颜色 for (int nIndex = 0; nIndex != PRN_CMYKOrRBLk; ++nIndex) { if (g_nColorIndexOfCMYKOrRBLk[nIndex] == nColorIndex) { stuCleanSprayInkInfo->dInkCost[nIndex] += dColorCost; } } } else //其它颜色模式 { stuCleanSprayInkInfo->dInkCost[nColorIndex] += dColorCost; } } //清喷信息上报到MES stuCleanSprayInfo *pCleanSprayInfo = new stuCleanSprayInfo; pCleanSprayInfo->nRunTime = nCleanSprayTime; pCleanSprayInfo->strStartTime = QDateTime::fromTime_t(stuCleanSprayInkInfo->nStartTime).toString("yyyy-MM-dd hh:mm:ss"); pCleanSprayInfo->strEndTime = QDateTime::fromTime_t(stuCleanSprayInkInfo->nEndTime).toString("yyyy-MM-dd hh:mm:ss"); memcpy((char*)pCleanSprayInfo->dInkCost, (char*)stuCleanSprayInkInfo->dInkCost, sizeof(double) * MAXCOLORS); emit(signal_SendMes(MES_MSG_CLEANSPRAY, pCleanSprayInfo)); emit(signal_AddInkInfo(CLEANSPRAY_INK_CALCULATE, stuCleanSprayInkInfo)); }
时间: 2024-02-21 22:00:34 浏览: 65
首先,可以使用智能指针来管理 `_CLEANSPRAY_INKINFO` 和 `stuCleanSprayInfo` 的内存,避免手动管理内存的问题。
其次,可以将一些重复的计算结果提前计算出来,避免重复计算。例如 `nCleanTotalTimes` 和 `dColorCost` 可以在循环之前计算出来。
最后,在循环中可以使用局部变量来存储一些重复计算的结果,避免多次调用函数。例如在循环中多次调用 `m_qPrintParam->GetCntOfColors()` 可以在循环之前将其计算出来并存储在变量中。
下面是优化后的代码示例:
```cpp
void QQuickPrint::CalcCleanSprayInk(int nCleanSprayTime, int nCleanSprayStartTime, int nCleanSprayEndTime) {
if (nCleanSprayTime <= 0)
return;
auto stuCleanSprayInkInfo = std::make_shared<_CLEANSPRAY_INKINFO>();
stuCleanSprayInkInfo->nCostTime = nCleanSprayTime;
stuCleanSprayInkInfo->nStartTime = static_cast<uint>(nCleanSprayStartTime);
stuCleanSprayInkInfo->nEndTime = static_cast<uint>(nCleanSprayEndTime);
stuCleanSprayInkInfo->nType = CLEANSPRAY_INK_CALCULATE;
const int nCntOfChannel = m_qPrintParam->GetCntOfChannel();
const int nFrameSize = m_qPrintParam->GetFrameSize();
const int nCleanDropSize = m_qPrintParam->GetCleanFireDropSize();
const double dDropSizeCost = CLEANSPRAYDROPSIZE[nCleanDropSize];
const int nCleanFireTimes = m_qPrintParam->GetCleanFireTimes();
const int nCleanFireInterval = m_qPrintParam->GetCleanFireInterval();
const int nCleanTotalTimes = (nCleanSprayTime / nCleanFireInterval) + 1;
const double dColorCost = PL2ML(nFrameSize * dDropSizeCost * nCleanFireTimes * nCleanTotalTimes);
std::vector<int> nChannelColors(nCntOfChannel);
for (int iC = 0; iC != nCntOfChannel; ++iC)
nChannelColors[iC] = m_qPrintParam->GetRIPDataOfPiece(iC);
for (int iC = 0; iC != nCntOfChannel; ++iC) {
const int nColorsCnt = m_qPrintParam->GetCntOfColors();
const int nColorIndex = nChannelColors[iC];
if (PRN_CMYKOrRBLk == nColorsCnt) {
for (int nIndex = 0; nIndex != PRN_CMYKOrRBLk; ++nIndex) {
if (g_nColorIndexOfCMYKOrRBLk[nIndex] == nColorIndex) {
stuCleanSprayInkInfo->dInkCost[nIndex] += dColorCost;
}
}
} else {
stuCleanSprayInkInfo->dInkCost[nColorIndex] += dColorCost;
}
}
auto pCleanSprayInfo = std::make_shared<stuCleanSprayInfo>();
pCleanSprayInfo->nRunTime = nCleanSprayTime;
pCleanSprayInfo->strStartTime = QDateTime::fromTime_t(stuCleanSprayInkInfo->nStartTime).toString("yyyy-MM-dd hh:mm:ss");
pCleanSprayInfo->strEndTime = QDateTime::fromTime_t(stuCleanSprayInkInfo->nEndTime).toString("yyyy-MM-dd hh:mm:ss");
memcpy((char*)pCleanSprayInfo->dInkCost, (char*)stuCleanSprayInkInfo->dInkCost, sizeof(double) * MAXCOLORS);
emit(signal_SendMes(MES_MSG_CLEANSPRAY, pCleanSprayInfo));
emit(signal_AddInkInfo(CLEANSPRAY_INK_CALCULATE, stuCleanSprayInkInfo));
}
```
阅读全文
相关推荐
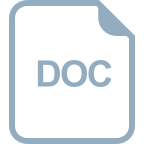
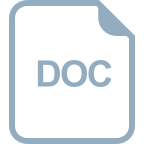
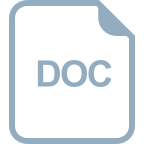
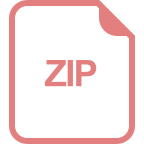
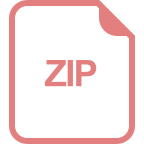
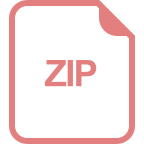
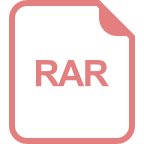
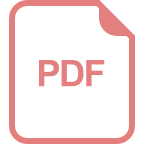
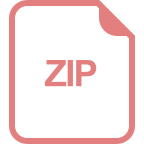
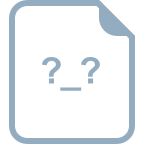
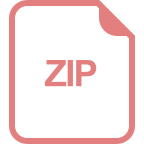
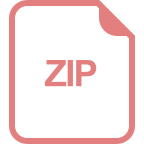
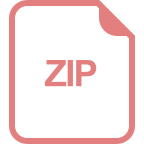
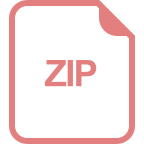
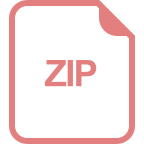
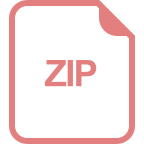
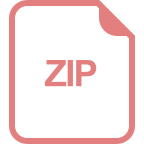
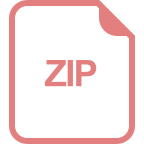
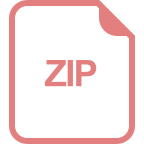