vue3.2 在ts下如何让props有默认值
时间: 2024-02-13 10:05:04 浏览: 29
在 Vue 3 中使用 TypeScript,可以通过以下方式为 props 设置默认值:
1. 首先在 Vue 组件中定义 props:
```typescript
import { defineComponent, PropType } from 'vue'
export default defineComponent({
name: 'MyComponent',
props: {
message: {
type: String as PropType<string>,
default: 'Hello world!'
}
},
// ...
})
```
2. 直接在 props 的类型定义中设置默认值:
```typescript
import { defineComponent, PropType } from 'vue'
export default defineComponent({
name: 'MyComponent',
props: {
message: {
type: String as PropType<string>,
default: 'Hello world!'
}
},
// ...
})
```
注意:需要使用 `PropType` 来定义 props 的类型,将默认值直接设置在 props 的类型定义中即可。这种方式不需要转换默认值的类型,也更加简洁明了。
相关问题
vue3.2在ts下如何让props有默认值
在 Vue 3 中使用 TypeScript,可以通过以下方式为 props 设置默认值:
1. 首先在 Vue 组件中定义 props:
```typescript
import { defineComponent, PropType } from 'vue'
export default defineComponent({
name: 'MyComponent',
props: {
message: {
type: String as PropType<string>,
default: 'Hello world!'
}
},
// ...
})
```
2. 直接在 props 的类型定义中设置默认值:
```typescript
import { defineComponent, PropType } from 'vue'
export default defineComponent({
name: 'MyComponent',
props: {
message: {
type: String as PropType<string>,
default: 'Hello world!'
}
},
// ...
})
```
注意:需要使用 `PropType` 来定义 props 的类型,将默认值直接设置在 props 的类型定义中即可。这种方式不需要转换默认值的类型,也更加简洁明了。
vue.2在ts下如何让props有默认值
在 Vue 2 中使用 TypeScript,可以通过以下方式为 props 设置默认值:
1. 首先在 Vue 组件中定义 props:
```typescript
export default Vue.extend({
name: 'MyComponent',
props: {
message: {
type: String,
default: 'Hello world!'
}
},
// ...
})
```
2. 在 TypeScript 中,需要使用 `PropOptions` 类型来定义 props 的默认值:
```typescript
import { PropOptions } from 'vue'
export default Vue.extend({
name: 'MyComponent',
props: {
message: {
type: String,
default: 'Hello world!' as PropOptions<string>['default']
}
},
// ...
})
```
注意:需要将默认值转换为对应类型的 `PropOptions` 中的 `default` 属性类型,以便 TypeScript 正确地推断 props 的类型。
相关推荐
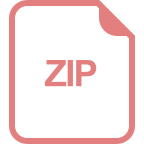












