解释代码const state = reactive({ inputText: "", isKeyboardVisible: false, messages: [ { content: "施主你好,很高兴为您服务!", msg: { time:"" } }, ], sendMessage: () => { const content = state.inputText.trim(); if (content) { const time = new Date(); const message = { id: state.messages.length + 1, content, time, isUser: true, avatar:'1' }; state.messages.push(message); state.inputText = ""; state.replyMessage(); } }, replyMessage: () => { const content = "尊敬的施主,您好。我们已收到您的咨询,请稍后。"; const time = new Date(); const message = { id: state.messages.length + 1, content, time, isUser: false, avatar:'2' }; setTimeout(() => { state.messages.push(message); }, 1000); }, formatDate: (time) => { const date = time?new Date(time):new Date(); const hours = date.getHours().toString().padStart(2, "0"); const minutes = date.getMinutes().toString().padStart(2, "0"); return `${hours}:${minutes}`; }, showKeyboard() { state.isKeyboardVisible = true; document.querySelector("input").focus(); }, hideKeyboard() { state.isKeyboardVisible = false; document.activeElement.focus(); }, });
时间: 2024-02-14 15:20:23 浏览: 33
这段代码使用了 Vue 3 中的响应式 API,创建了一个名为 state 的对象,包含了一些属性和方法:
- inputText: 输入框中的文本内容;
- isKeyboardVisible: 是否显示虚拟键盘;
- messages: 聊天记录,初始值为一条欢迎消息;
- sendMessage: 发送消息的方法,会将输入框中的文本内容作为一条用户消息加入聊天记录中,并调用 replyMessage 方法生成一条机器人回复消息;
- replyMessage: 生成机器人回复消息的方法,会在 1 秒后将一条默认回复消息加入聊天记录中;
- formatDate: 将时间戳格式化为 hh:mm 的方法;
- showKeyboard: 显示虚拟键盘的方法,将 isKeyboardVisible 设为 true 并使输入框获得焦点;
- hideKeyboard: 隐藏虚拟键盘的方法,将 isKeyboardVisible 设为 false 并使输入框失去焦点。
这段代码的作用是创建一个简单的聊天界面,用户可以在输入框中输入消息,机器人会自动回复默认消息,并将聊天记录显示在界面上。其中使用了 Vue 3 的响应式 API,可以方便地更新界面上的数据。
相关问题
const eleNode = reactive({}) const obj = { name: 'A', type: 'input' } eleNode = obj
这段代码有问题,因为你不能对 `reactive` 的对象进行重新赋值。如果你想改变 `eleNode` 中的属性值,应该直接对属性进行修改,例如 `eleNode.name = 'B'`。如果你想将一个普通对象转化为响应式对象,可以使用 `toRefs` 方法,例如:
```
const eleNode = reactive({})
const obj = {
name: 'A',
type: 'input'
}
Object.assign(eleNode, toRefs(obj))
```
这样就可以将 `obj` 中的属性转化为响应式属性,并添加到 `eleNode` 中。注意,这里使用了 `Object.assign` 将 `toRefs(obj)` 的属性合并到了 `eleNode` 中。现在你可以通过 `eleNode.name` 来访问 `obj` 中的 `name` 属性,并且任何对 `eleNode.name` 的修改都会触发响应式更新。
<script setup> import useGetUrl from "@/hooks/useGetUrl"; import { reactive ,onMounted} from "vue"; const state = reactive({ inputText: "", isKeyboardVisible: false, messages: [ { content: "施主你好,很高兴为您服务!", msg: { time:"" } }, ], sendMessage: () => { const content = state.inputText.trim(); if (content) { const time = new Date(); const message = { id: state.messages.length + 1, content, time, isUser: true, }; state.messages.push(message); state.inputText = ""; state.replyMessage(); } }, replyMessage: () => { const content = "尊敬的施主,您好。我们已收到您的咨询,请稍后。"; const time = new Date(); const message = { id: state.messages.length + 1, content, time, isUser: false, }; setTimeout(() => { state.messages.push(message); }, 1000); }, formatDate: (time) => { const date = time?new Date(time):new Date(); const hours = date.getHours().toString().padStart(2, "0"); const minutes = date.getMinutes().toString().padStart(2, "0"); return ${hours}:${minutes}; }, showKeyboard() { state.isKeyboardVisible = true; document.querySelector("input").focus(); }, hideKeyboard() { state.isKeyboardVisible = false; document.activeElement.focus(); }, // handleScroll() { // const chatList = this.$refs.chatList; // const scrollTop = chatList.scrollTop; // const scrollHeight = chatList.scrollHeight; // const clientHeight = chatList.clientHeight; // if (scrollTop === 0) { // // 聊天列表滚动到了顶部,需要加载更多聊天记录 // // TODO: 加载更多聊天记录 // // 滚动到之前的位置,避免列表跳动 // chatList.scrollTop = chatList.scrollHeight - scrollHeight; // } else if (scrollTop + clientHeight >= scrollHeight) { // // 聊天列表滚动到了底部,需要将列表自动上滑 // chatList.scrollTop = chatList.scrollHeight - clientHeight; // } // }, }); onMounted(() => { state.messages[0].msg.time = state.formatDate() }) </script>在该段代码中增加列表置底后上滑
可以在聊天列表上添加一个ref属性,用于获取聊天列表的dom节点,然后在sendMessage和replyMessage方法中,将聊天列表的scrollTop设置为聊天列表的scrollHeight,实现自动置底。在handleScroll方法中,可以判断scrollTop是否为0,如果为0则说明聊天列表已经滚动到了顶部,可以加载更多聊天记录;如果scrollTop加上clientHeight大于等于scrollHeight,则说明聊天列表已经滚动到了底部,需要将列表自动上滑。以下是修改后的代码:
```
<template>
<div class="chat-container">
<div class="chat-header">在线客服</div>
<div class="chat-list" ref="chatList">
<div class="chat-message" v-for="message in state.messages" :key="message.id">
<div class="chat-content" :class="{'user': message.isUser}">
<div class="chat-text">{{ message.content }}</div>
<div class="chat-time">{{ state.formatDate(message.time) }}</div>
</div>
</div>
</div>
<div class="chat-input">
<div class="chat-input-box" :class="{'show-keyboard': state.isKeyboardVisible}">
<input type="text" v-model="state.inputText" placeholder="请输入您的问题" />
<div class="chat-input-btn" @click="state.sendMessage">发送</div>
<div class="chat-input-keyboard" @click="state.showKeyboard">键盘</div>
<div class="chat-input-voice">语音</div>
</div>
<div class="chat-input-keyboard-box" v-show="state.isKeyboardVisible">
<div class="chat-input-keyboard-item" v-for="(item, index) in state.keyboard" :key="index" @click="state.inputText += item">{{ item }}</div>
<div class="chat-input-keyboard-item" @click="state.inputText = state.inputText.slice(0, -1)">删除</div>
<div class="chat-input-keyboard-item" @click="state.hideKeyboard">收起</div>
</div>
</div>
</div>
</template>
<script setup>
import { reactive, onMounted } from "vue";
import useGetUrl from "@/hooks/useGetUrl";
const state = reactive({
inputText: "",
isKeyboardVisible: false,
messages: [
{
content: "施主你好,很高兴为您服务!",
time: new Date(),
isUser: false,
},
],
keyboard: ["1", "2", "3", "4", "5", "6", "7", "8", "9", "0"],
sendMessage: () => {
const content = state.inputText.trim();
if (content) {
const time = new Date();
const message = {
id: state.messages.length + 1,
content,
time,
isUser: true,
};
state.messages.push(message);
state.inputText = "";
state.replyMessage();
state.scrollToBottom();
}
},
replyMessage: () => {
const content = "尊敬的施主,您好。我们已收到您的咨询,请稍后。";
const time = new Date();
const message = {
id: state.messages.length + 1,
content,
time,
isUser: false,
};
setTimeout(() => {
state.messages.push(message);
state.scrollToBottom();
}, 1000);
},
formatDate: (time) => {
const date = time ? new Date(time) : new Date();
const hours = date.getHours().toString().padStart(2, "0");
const minutes = date.getMinutes().toString().padStart(2, "0");
return `${hours}:${minutes}`;
},
showKeyboard() {
state.isKeyboardVisible = true;
document.querySelector("input").focus();
},
hideKeyboard() {
state.isKeyboardVisible = false;
document.activeElement.focus();
},
scrollToBottom() {
const chatList = this.$refs.chatList;
chatList.scrollTop = chatList.scrollHeight;
},
});
onMounted(() => {
state.messages[0].time = state.formatDate();
});
</script>
<style scoped>
.chat-container {
width: 100%;
height: 100%;
display: flex;
flex-direction: column;
}
.chat-header {
height: 40px;
line-height: 40px;
background-color: #f5f5f5;
text-align: center;
}
.chat-list {
flex: 1;
overflow-y: auto;
padding: 10px;
}
.chat-message {
margin-bottom: 10px;
}
.chat-content {
display: inline-block;
padding: 5px 10px;
border-radius: 10px;
max-width: 80%;
word-wrap: break-word;
}
.chat-content.user {
text-align: right;
background-color: #e6f7ff;
}
.chat-content.user .chat-text {
display: inline-block;
text-align: left;
}
.chat-content:not(.user) {
text-align: left;
background-color: #fff;
}
.chat-time {
font-size: 12px;
color: #999;
text-align: right;
margin-top: 5px;
}
.chat-input {
height: 50px;
border-top: 1px solid #ddd;
display: flex;
align-items: center;
justify-content: center;
position: relative;
}
.chat-input-box {
width: 100%;
display: flex;
align-items: center;
justify-content: center;
position: absolute;
bottom: 0;
left: 0;
padding: 5px;
}
.chat-input-btn {
background-color: #409eff;
color: #fff;
padding: 5px 10px;
border-radius: 5px;
margin-left: 10px;
cursor: pointer;
}
.chat-input-keyboard {
font-size: 20px;
margin-left: 10px;
cursor: pointer;
}
.chat-input-voice {
font-size: 20px;
margin-left: 10px;
cursor: pointer;
}
.chat-input-keyboard-box {
width: 100%;
display: none;
position: absolute;
bottom: 50px;
left: 0;
background-color: #fff;
border-top: 1px solid #ddd;
}
.chat-input-keyboard-box.show {
display: block;
}
.chat-input-keyboard-item {
display: inline-block;
width: calc(100% / 3);
height: 40px;
line-height: 40px;
text-align: center;
cursor: pointer;
}
.chat-input-keyboard-item:nth-child(10) {
width: calc(100% / 3 * 2);
}
.show-keyboard .chat-input-keyboard-box {
display: block;
}
</style>
```
相关推荐
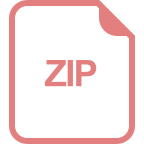
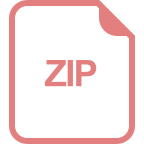
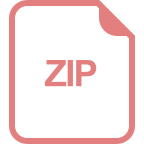












