不用到localdate函数
时间: 2024-10-14 14:07:51 浏览: 22
如果你想避免直接使用`LocalDate`,可以创建一个包含月份和天数的数据结构来模拟用户的生日,然后通过比较两个结构来进行猜测。以下是一个简化版的程序示例:
```java
import java.util.Scanner;
class BirthdayGuess {
private int year;
private int month;
private int day;
public BirthdayGuess(int year, int month, int day) {
this.year = year;
this.month = month;
this.day = day;
}
public boolean isBefore(BirthdayGuess other) {
int myTotalDays = getNumberOfDaysInMonth(month, year) + day - 1;
int theirTotalDays = getNumberOfDaysInMonth(other.month, other.year) + other.day - 1;
return myTotalDays < theirTotalDays;
}
// 自己计算每个月的天数,这里为了简单只考虑非闰年的规则
private int getNumberOfDaysInMonth(int month, int year) {
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
default: // February
if ((year % 4 != 0 && year % 100 != 0) || (year % 400 == 0)) { // 非闰年
return 28;
} else { // 闰年
return 29;
}
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 用户输入生日信息
System.out.println("请输入你的出生年份、月份和日子,用空格分隔,例如2000 06 15:");
String[] birthdayParts = scanner.nextLine().split(" ");
BirthdayGuess userBirth = new BirthdayGuess(Integer.parseInt(birthdayParts[0]), Integer.parseInt(birthdayParts[1]), Integer.parseInt(birthdayParts[2]));
// 猜测直到正确
BirthdayGuess computerGuess = new BirthdayGuess(1900, 1, 1); // 假设从1900年开始
while (true) {
if (userBirth.isBefore(computerGuess)) {
System.out.println("恭喜!你的生日比我的早一些。请继续猜测。");
computerGuess = incrementMonth(computerGuess);
} else if (userBirth.isAfter(computerGuess)) {
System.out.println("哎呀,有点儿晚了哦!你的生日比我晚一些。请继续猜测。");
userBirth = incrementMonth(userBirth);
} else {
System.out.println("哇!我猜对了!你的生日是:" + userBirth);
break;
}
}
}
// 单月加一
private static BirthdayGuess incrementMonth(BirthdayGuess birthday) {
int oldMonth = birthday.month;
int daysInNewMonth = getNumberOfDaysInMonth(oldMonth + 1, birthday.year);
birthday.month++;
if (birthday.month > 12) {
birthday.month = 1;
birthday.year++;
}
birthday.day = Math.min(daysInNewMonth, birthday.day + 1);
return birthday;
}
}
```
这个版本的程序不再依赖`LocalDate`类,而是通过自定义的生日结构和一些辅助函数实现了猜测过程。
阅读全文
相关推荐
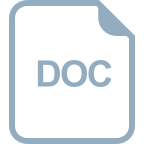
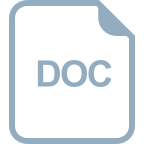
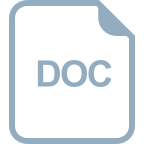















