使用Arduino +lvgl+esp32+tft_espi写一个网络天气时钟
时间: 2023-05-22 18:02:29 浏览: 984
很高兴回答你的问题,以下是使用Arduino、lvgl、esp32和tft_espi的示例代码实现网络天气时钟的方法:
```
#include <Arduino.h>
#include <TFT_eSPI.h>
#include <lvgl.h>
#include <WiFi.h>
#include <ArduinoJson.h>
#include <time.h>
#define WIFI_SSID "your_wifi_ssid"
#define WIFI_PASS "your_wifi_password"
#define OPENWEATHERMAP_APIKEY "your_openweathermap_api_key"
TFT_eSPI tft = TFT_eSPI(135, 240); // 设置 TFT 屏幕的大小
lv_obj_t * label_temperature, * label_temperature_symbol, * label_weather_description, * label_city_name, * label_clock;
void get_weather_data(String & temperature, String & weather_description, String & city_name) {
WiFiClient client;
if (!client.connect("api.openweathermap.org", 80)) {
Serial.println("connection failed!");
return;
}
String url = "/data/2.5/weather?q=Shenzhen,CN&appid=" OPENWEATHERMAP_APIKEY "&units=metric";
client.print(String("GET ") + url + " HTTP/1.1\r\n" +
"Host: api.openweathermap.org\r\n" +
"Connection: close\r\n\r\n");
String response = "";
while (client.connected()) {
String line = client.readStringUntil('\n');
if (line == "\r") {
break;
}
}
while (client.available()) {
response += (char)client.read();
}
const int capacity = JSON_OBJECT_SIZE(4) + 100;
DynamicJsonDocument doc(capacity);
deserializeJson(doc, response);
JsonObject main = doc["main"];
temperature = main["temp"].as<String>();
JsonArray weather = doc["weather"];
weather_description = weather[0]["description"].as<String>();
city_name = doc["name"].as<String>();
}
void get_time_string(String & time_string) {
time_t now;
struct tm timeinfo;
time(&now);
localtime_r(&now, &timeinfo);
char strftime_buf[64];
strftime(strftime_buf, sizeof(strftime_buf), "%H:%M", &timeinfo);
time_string = strftime_buf;
}
void setup() {
Serial.begin(115200);
lv_init();
tft.init();
tft.setRotation(3);
tft.fillScreen(TFT_BLACK);
lvgl_driver_init(&tft);
lv_disp_drv_t disp_drv;
lv_disp_drv_init(&disp_drv);
disp_drv.buffer = &lvgl_buffer;
disp_drv.flush_cb = lvgl_flush;
lv_disp_drv_register(&disp_drv);
lv_theme_t * theme = lv_theme_material_init(210, NULL);
lv_theme_set_current(theme);
label_temperature = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(label_temperature, "temperature");
lv_obj_align(label_temperature, NULL, LV_ALIGN_CENTER, 0, -40);
label_temperature_symbol = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(label_temperature_symbol, "°C");
lv_obj_set_pos(label_temperature_symbol, lv_obj_get_x(label_temperature) + lv_obj_get_width(label_temperature), lv_obj_get_y(label_temperature));
label_weather_description = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(label_weather_description, "weather_description");
lv_obj_align(label_weather_description, NULL, LV_ALIGN_CENTER, 0, 0);
label_city_name = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(label_city_name, "city_name");
lv_obj_align(label_city_name, NULL, LV_ALIGN_CENTER, 0, 40);
label_clock = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(label_clock, "clock");
lv_obj_align(label_clock, NULL, LV_ALIGN_CENTER, 0, 80);
lv_task_create([](lv_task_t * task) {
String temperature;
String weather_description;
String city_name;
get_weather_data(temperature, weather_description, city_name);
lv_label_set_text(label_temperature, temperature.c_str());
lv_label_set_text(label_weather_description, weather_description.c_str());
lv_label_set_text(label_city_name, city_name.c_str());
}, 5000, LV_TASK_PRIO_LOW, NULL);
lv_task_create([](lv_task_t * task) {
String time_string;
get_time_string(time_string);
lv_label_set_text(label_clock, time_string.c_str());
}, 1000, LV_TASK_PRIO_MID, NULL);
WiFi.begin(WIFI_SSID, WIFI_PASS);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
}
Serial.println("setup complete!");
}
void loop() {
lv_task_handler();
delay(1);
}
```
该代码使用了 OpenWeatherMap API 获取深圳的当前天气数据,在屏幕上输出温度、天气描述和城市名称,通过 `lv_task_create` 函数设置定时任务每 5 秒钟更新一次天气信息和每秒钟更新一次当前时间,是一个简单而实用的网络天气时钟的实现方式。
注:这里使用的API Key只是做演示,请勿用于商业用途,如有需要请自行注册。
阅读全文
相关推荐

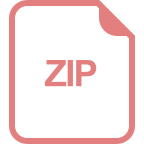
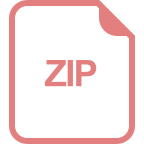
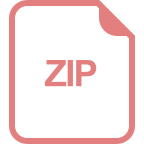
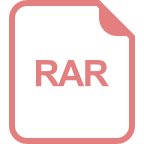
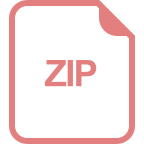
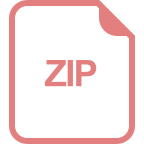
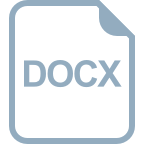
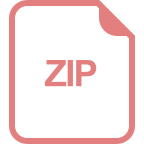
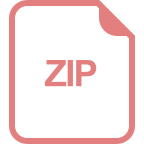
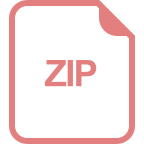
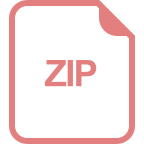
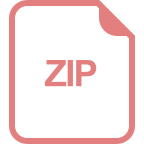
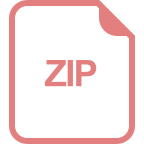