java生成docx_JAVA通过模板生成DOCX文档
时间: 2023-09-17 09:07:21 浏览: 50
可以使用Apache POI和FreeMarker来实现Java生成docx文档的功能。以下是一个简单的示例,演示如何使用这两个库来生成docx文档。
1. 首先,你需要在项目中导入Apache POI和FreeMarker的依赖。这可以在pom.xml文件中完成,如下所示:
```
<dependencies>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.30</version>
</dependency>
</dependencies>
```
2. 创建一个docx模板文件。你可以使用Microsoft Word等工具来创建模板文件,并将其保存为docx格式。
3. 在Java中编写代码来读取模板文件,并将数据填充到模板中。以下是一个示例代码:
```java
import java.io.*;
import java.util.*;
import org.apache.poi.xwpf.usermodel.*;
import org.apache.poi.openxml4j.exceptions.InvalidFormatException;
import org.apache.poi.util.Units;
import freemarker.template.Configuration;
import freemarker.template.Template;
public class DocxGenerator {
public static void main(String[] args) {
Map<String, Object> data = new HashMap<>();
data.put("name", "John Doe");
data.put("age", 30);
data.put("address", "123 Main St.");
try {
// Read the template file
InputStream is = new FileInputStream("template.docx");
XWPFDocument doc = new XWPFDocument(is);
// Use FreeMarker to populate the template with data
Configuration cfg = new Configuration(Configuration.VERSION_2_3_30);
cfg.setClassForTemplateLoading(DocxGenerator.class, "/");
Template template = cfg.getTemplate("template.ftl");
StringWriter writer = new StringWriter();
template.process(data, writer);
// Replace the placeholder in the document with the generated content
for (XWPFParagraph p : doc.getParagraphs()) {
List<XWPFRun> runs = p.getRuns();
if (runs != null) {
for (XWPFRun r : runs) {
String text = r.getText(0);
if (text != null && text.contains("{{content}}")) {
text = text.replace("{{content}}", writer.toString());
r.setText(text, 0);
}
}
}
}
// Save the document to a file
OutputStream os = new FileOutputStream("output.docx");
doc.write(os);
os.close();
doc.close();
} catch (IOException e) {
e.printStackTrace();
} catch (InvalidFormatException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们首先从模板文件中读取docx文档,并使用FreeMarker将数据填充到模板中。然后,我们遍历文档中的段落和运行,并查找包含“{{content}}”的文本,并将其替换为生成的内容。最后,我们将生成的文档保存到一个新文件中。
4. 创建一个FreeMarker模板文件,以指定如何填充数据。以下是一个示例模板文件:
```
Name: {{name}}
Age: {{age}}
Address: {{address}}
```
在这个模板中,我们使用“{{name}}”、“{{age}}”和“{{address}}”作为占位符,以指定生成的文档中的数据位置。
5. 运行Java代码,生成docx文档。
这是一个简单的示例,演示了如何使用Java生成docx文档。你可以根据自己的需求修改代码和模板文件,以生成更复杂的文档。
相关推荐
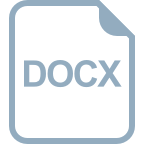
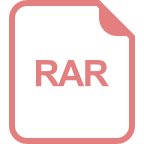
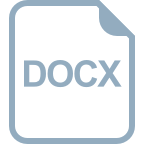














