将convlstm加到YOLOv7骨干网络中,写pytorch代码
时间: 2024-02-26 13:55:05 浏览: 89
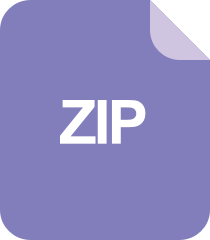
convnext的代码-pytorch框架-cv中可以使用
好的,以下是将ConvLSTM加到YOLOv7骨干网络中的PyTorch代码。请注意,这仅是一个示例代码,您需要根据自己的需求进行修改和调整。
首先,我们需要导入所需的库和模块:
``` python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variable
```
接下来,我们定义ConvLSTM模块:
``` python
class ConvLSTM(nn.Module):
def __init__(self, in_channels, hidden_channels, kernel_size, batch_size, bias=True):
super(ConvLSTM, self).__init__()
self.input_dim = in_channels
self.hidden_dim = hidden_channels
self.kernel_size = kernel_size
self.bias = bias
self.batch_size = batch_size
self.padding = int((kernel_size - 1) / 2)
self.conv = nn.Conv2d(in_channels=self.input_dim + self.hidden_dim,
out_channels=4 * self.hidden_dim,
kernel_size=self.kernel_size,
padding=self.padding,
bias=self.bias)
def forward(self, input_tensor, cur_state):
h_cur, c_cur = cur_state
combined = torch.cat([input_tensor, h_cur], dim=1)
combined_conv = self.conv(combined)
cc_i, cc_f, cc_o, cc_g = torch.split(combined_conv, self.hidden_dim, dim=1)
i = torch.sigmoid(cc_i)
f = torch.sigmoid(cc_f)
o = torch.sigmoid(cc_o)
g = torch.tanh(cc_g)
c_next = f * c_cur + i * g
h_next = o * torch.tanh(c_next)
return h_next, c_next
def init_hidden(self):
return (Variable(torch.zeros(self.batch_size, self.hidden_dim, 7, 7)).cuda(),
Variable(torch.zeros(self.batch_size, self.hidden_dim, 7, 7)).cuda())
```
然后,我们定义包含ConvLSTM的YOLOv7骨干网络:
``` python
class YOLOv7_ConvLSTM(nn.Module):
def __init__(self):
super(YOLOv7_ConvLSTM, self).__init__()
self.conv1 = nn.Conv2d(3, 32, 3, stride=1, padding=1, bias=False)
self.bn1 = nn.BatchNorm2d(32)
self.conv2 = nn.Conv2d(32, 64, 3, stride=2, padding=1, bias=False)
self.bn2 = nn.BatchNorm2d(64)
self.conv3 = nn.Conv2d(64, 32, 1, stride=1, padding=0, bias=False)
self.bn3 = nn.BatchNorm2d(32)
self.conv4 = nn.Conv2d(32, 64, 3, stride=2, padding=1, bias=False)
self.bn4 = nn.BatchNorm2d(64)
self.conv5 = nn.Conv2d(64, 32, 1, stride=1, padding=0, bias=False)
self.bn5 = nn.BatchNorm2d(32)
self.conv_lstm = ConvLSTM(in_channels=32, hidden_channels=32, kernel_size=3, batch_size=1)
def forward(self, x):
h, w = x.size()[2:]
x = F.leaky_relu(self.bn1(self.conv1(x)), 0.1, inplace=True)
x = F.leaky_relu(self.bn2(self.conv2(x)), 0.1, inplace=True)
x = F.leaky_relu(self.bn3(self.conv3(x)), 0.1, inplace=True)
x = F.leaky_relu(self.bn4(self.conv4(x)), 0.1, inplace=True)
x = F.leaky_relu(self.bn5(self.conv5(x)), 0.1, inplace=True)
x = x.view(-1, 32, h // 16, w // 16)
h, c = self.conv_lstm(x, self.conv_lstm.init_hidden())
return h
```
在这里,我们使用了YOLOv7的骨干网络,同时还添加了一个包含ConvLSTM的模块。在前向传递中,我们首先对输入图像进行标准卷积操作,然后将输出的张量重塑成适合ConvLSTM模块的形状,并将其输入到ConvLSTM模块中。最后,我们仅返回ConvLSTM的输出。
这是一个简单的示例代码,您可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
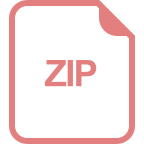
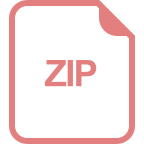
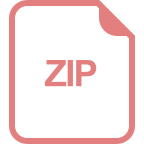
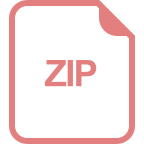
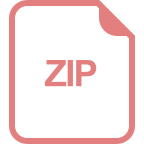
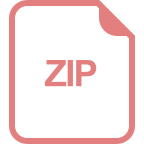
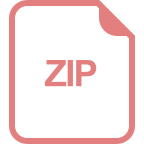
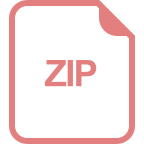
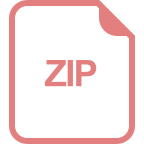
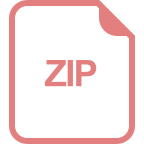
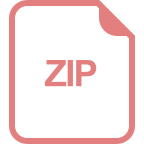
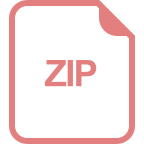
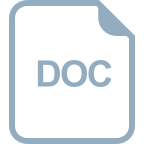
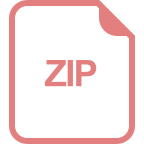
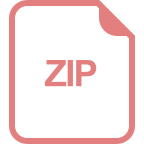
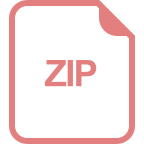