九宫格抽奖带跑马灯代码实现
时间: 2023-08-25 09:05:51 浏览: 42
以下是一种可能的九宫格抽奖带跑马灯的代码实现:
HTML代码:
```
<div class="lottery-container">
<div class="lottery-grid">
<div class="lottery-cell" data-index="0">1</div>
<div class="lottery-cell" data-index="1">2</div>
<div class="lottery-cell" data-index="2">3</div>
<div class="lottery-cell" data-index="3">4</div>
<div class="lottery-cell" data-index="4">5</div>
<div class="lottery-cell" data-index="5">6</div>
<div class="lottery-cell" data-index="6">7</div>
<div class="lottery-cell" data-index="7">8</div>
<div class="lottery-cell" data-index="8">9</div>
</div>
<div class="lottery-result">恭喜您获得<span class="result-text"></span></div>
<div class="lottery-marquee">
<div class="marquee-list">
<div class="marquee-item">恭喜用户A获得了奖品1!</div>
<div class="marquee-item">恭喜用户B获得了奖品2!</div>
<div class="marquee-item">恭喜用户C获得了奖品3!</div>
<div class="marquee-item">恭喜用户D获得了奖品4!</div>
<div class="marquee-item">恭喜用户E获得了奖品5!</div>
<div class="marquee-item">恭喜用户F获得了奖品6!</div>
<div class="marquee-item">恭喜用户G获得了奖品7!</div>
<div class="marquee-item">恭喜用户H获得了奖品8!</div>
<div class="marquee-item">恭喜用户I获得了奖品9!</div>
</div>
</div>
<div class="lottery-button">开始抽奖</div>
</div>
```
CSS代码:
```
.lottery-container {
position: relative;
width: 300px;
height: 300px;
margin: 0 auto;
font-size: 24px;
text-align: center;
}
.lottery-grid {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
width: 100%;
height: 100%;
background-color: #f2f2f2;
}
.lottery-cell {
display: flex;
justify-content: center;
align-items: center;
width: 32%;
height: 32%;
border: 1px solid #ccc;
}
.lottery-result {
position: absolute;
top: 10px;
left: 50%;
transform: translateX(-50%);
width: 80%;
height: 40px;
line-height: 40px;
background-color: #fff;
border: 1px solid #ccc;
}
.result-text {
font-weight: bold;
color: red;
}
.lottery-marquee {
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
width: 80%;
height: 40px;
line-height: 40px;
overflow: hidden;
}
.marquee-list {
display: flex;
width: 200%;
animation: marquee 10s linear infinite;
}
.marquee-item {
width: 50%;
}
.lottery-button {
position: absolute;
bottom: 50%;
left: 50%;
transform: translate(-50%, 50%);
width: 120px;
height: 50px;
line-height: 50px;
background-color: #ff5722;
color: #fff;
border-radius: 25px;
cursor: pointer;
}
.lottery-button:hover {
background-color: #ff7043;
}
@keyframes marquee {
0% {
transform: translateX(0%);
}
100% {
transform: translateX(-50%);
}
}
```
JavaScript代码:
```
const grid = document.querySelector('.lottery-grid');
const cells = document.querySelectorAll('.lottery-cell');
const result = document.querySelector('.result-text');
const marquee = document.querySelector('.marquee-list');
const button = document.querySelector('.lottery-button');
let index = -1;
let timer = null;
function startLottery() {
clearInterval(timer);
index = -1;
timer = setInterval(() => {
// 取消上一个选中的格子的样式
if (index >= 0) {
cells[index].classList.remove('selected');
}
// 随机选中一个格子
index = Math.floor(Math.random() * cells.length);
cells[index].classList.add('selected');
}, 100);
}
function stopLottery() {
clearInterval(timer);
result.textContent = cells[index].textContent;
cells[index].classList.remove('selected');
marquee.insertBefore(document.createElement('div'), marquee.firstChild).textContent = `恭喜用户${Math.random().toString(36).substring(2)}获得了${result.textContent}!`;
}
button.addEventListener('click', () => {
if (timer) {
stopLottery();
} else {
startLottery();
}
});
```
说明:
- HTML中的九宫格使用flex布局实现,每个格子使用data-index属性标记其在数组中的位置。
- CSS中的跑马灯使用CSS动画实现,滚动的速度和时间通过animation属性控制。
- JavaScript中的抽奖过程使用setInterval实现,每隔一定时间随机选中一个格子,并添加选中样式。停止抽奖时,清除定时器,显示中奖结果,取消选中样式,并在跑马灯中添加一条中奖信息,其中用户名随机生成。
相关推荐










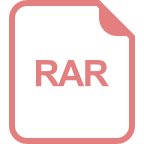