用python编程实现小波系数提取
时间: 2023-09-26 08:08:59 浏览: 31
小波变换是一种信号分析技术,可以将信号分解成不同频率的子信号并进行重构。小波系数提取是指从小波变换后的子信号中提取有用的信息,通常是频率、振幅或相位等方面的特征。
以下是使用Python实现小波系数提取的示例代码:
```python
import pywt
import numpy as np
# 生成测试信号
x = np.linspace(0, 2*np.pi, num=256)
y = np.sin(32*x) + np.sin(64*x)
# 进行小波变换
coeffs = pywt.wavedec(y, 'db4', level=5)
# 提取近似系数和细节系数
cA5, cD5, cD4, cD3, cD2, cD1 = coeffs
# 对细节系数进行能量归一化
cD5 /= np.sqrt(2)
cD4 /= np.sqrt(2**2)
cD3 /= np.sqrt(2**3)
cD2 /= np.sqrt(2**4)
cD1 /= np.sqrt(2**5)
# 绘制小波系数分解图像
import matplotlib.pyplot as plt
plt.figure(figsize=(8,6))
plt.subplot(6,1,1)
plt.plot(cA5)
plt.title('Approximation coefficients')
plt.subplot(6,1,2)
plt.plot(cD5)
plt.title('Level 5 detail coefficients')
plt.subplot(6,1,3)
plt.plot(cD4)
plt.title('Level 4 detail coefficients')
plt.subplot(6,1,4)
plt.plot(cD3)
plt.title('Level 3 detail coefficients')
plt.subplot(6,1,5)
plt.plot(cD2)
plt.title('Level 2 detail coefficients')
plt.subplot(6,1,6)
plt.plot(cD1)
plt.title('Level 1 detail coefficients')
plt.tight_layout()
plt.show()
```
在上述代码中,我们使用了Python的pywt库进行小波变换,并使用db4小波基对信号进行了5层小波分解,得到了6个子信号(近似系数和5层细节系数)。然后对5层细节系数进行了能量归一化处理,并绘制出了各层小波系数的图像。
阅读全文
相关推荐
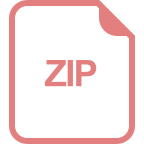

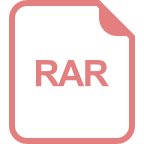
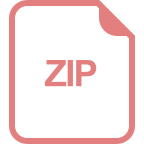
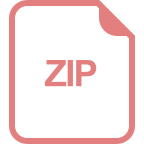
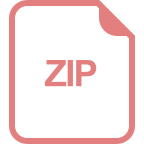
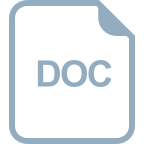
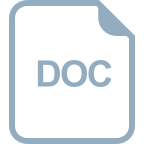


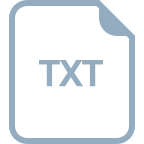
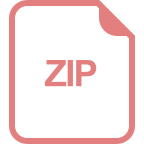
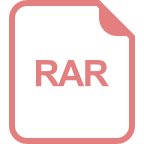
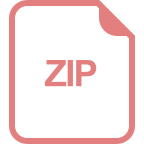
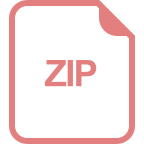
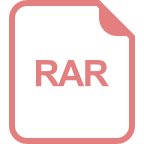
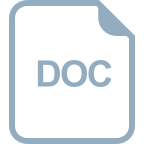