用QT读取MATLAB中mat文件中的Cell数据
时间: 2024-03-21 07:37:56 浏览: 14
可以使用MATLAB引擎库来读取MAT文件中的数据,然后将其转换为QT中的数据类型。以下是一个示例代码:
```c++
#include <QCoreApplication>
#include <QDebug>
#include <engine.h>
#include <matrix.h>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// Start MATLAB engine
Engine *ep;
if (!(ep = engOpen(""))) {
qDebug() << "Can't start MATLAB engine";
return EXIT_FAILURE;
}
// Read the mat file
mxArray *matData;
matData = engGetVariable(ep, "cellData");
// Get the number of cells in the cell array
int numCells = mxGetNumberOfElements(matData);
// Loop through each cell and extract the data
for (int i = 0; i < numCells; i++) {
mxArray *cell = mxGetCell(matData, i);
// Extract the data type and size of the cell contents
mxClassID dataType = mxGetClassID(cell);
const mwSize *dims = mxGetDimensions(cell);
int numRows = dims[0];
int numCols = dims[1];
// Extract the actual data
void *data = mxGetData(cell);
// Convert the data to a QT data type
if (dataType == mxDOUBLE_CLASS) {
double *doubleData = static_cast<double*>(data);
// Do something with the double data
qDebug() << "Double data for cell" << i << ":";
for (int row = 0; row < numRows; row++) {
for (int col = 0; col < numCols; col++) {
qDebug() << doubleData[row * numCols + col];
}
}
} else if (dataType == mxINT32_CLASS) {
int *intData = static_cast<int*>(data);
// Do something with the integer data
qDebug() << "Integer data for cell" << i << ":";
for (int row = 0; row < numRows; row++) {
for (int col = 0; col < numCols; col++) {
qDebug() << intData[row * numCols + col];
}
}
}
// Clean up the mxArray
mxDestroyArray(cell);
}
// Clean up the engine
engClose(ep);
return a.exec();
}
```
在上面的示例代码中,我们首先启动了MATLAB引擎,并使用`engGetVariable`函数从MAT文件中读取名为`cellData`的变量。然后,我们遍历每个单元格,提取其数据类型、大小和实际数据。最后,我们将数据转换为QT数据类型,并进行处理。
请注意,这只是一个示例代码,并且仅支持`mxDOUBLE_CLASS`和`mxINT32_CLASS`数据类型。如果您需要处理其他数据类型,请相应地修改代码。
相关推荐





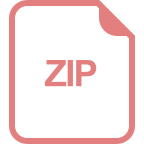
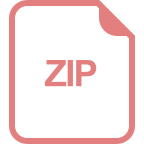
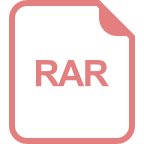