我构造一个程序,将kafka获取到的数据经过flink处理后保存在postgresql中,包括三个数据:时间戳、经度、纬度。同时构造一个无状态算子,即通过经纬度信息判断一个纬度29-30和经度116-117的范围内随着时间的增加有多少个对象出现在这个区域中,请帮我用ore.apache.flink构造完整的flink代码
时间: 2024-03-09 15:49:10 浏览: 18
好的,以下是一个可能的实现,其中假设 Kafka 中的数据以 JSON 格式发送,并且有一个名为 `input-topic` 的主题:
```java
import org.apache.flink.api.common.functions.MapFunction;
import org.apache.flink.api.common.serialization.SimpleStringSchema;
import org.apache.flink.api.java.functions.KeySelector;
import org.apache.flink.api.java.tuple.Tuple3;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.connectors.kafka.FlinkKafkaConsumer;
import org.apache.flink.streaming.connectors.kafka.FlinkKafkaProducer;
import org.apache.flink.streaming.util.serialization.JSONKeyValueDeserializationSchema;
import org.apache.flink.streaming.util.serialization.KeyedSerializationSchema;
import java.util.Properties;
public class KafkaFlinkPostgreSQLExample {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
// 设置 Kafka 消费者属性
Properties kafkaProps = new Properties();
kafkaProps.setProperty("bootstrap.servers", "localhost:9092");
kafkaProps.setProperty("group.id", "flink-group");
// 从 Kafka 中读取 JSON 数据
FlinkKafkaConsumer<String> kafkaConsumer = new FlinkKafkaConsumer<>(
"input-topic",
new SimpleStringSchema(),
kafkaProps
);
DataStream<Tuple3<Long, Double, Double>> inputStream = env
.addSource(kafkaConsumer)
.map(new MapFunction<String, Tuple3<Long, Double, Double>>() {
@Override
public Tuple3<Long, Double, Double> map(String value) throws Exception {
// 假设 JSON 数据格式为 { "timestamp": 1234567890, "longitude": 116.0, "latitude": 29.0 }
JSONObject json = new JSONObject(value);
long timestamp = json.getLong("timestamp");
double longitude = json.getDouble("longitude");
double latitude = json.getDouble("latitude");
return new Tuple3<>(timestamp, longitude, latitude);
}
});
// 利用 KeyBy 将数据流按照经纬度分组,并按照时间戳升序排序
DataStream<Tuple3<Long, Double, Double>> sortedStream = inputStream
.keyBy(new KeySelector<Tuple3<Long, Double, Double>, Tuple2<Double, Double>>() {
@Override
public Tuple2<Double, Double> getKey(Tuple3<Long, Double, Double> value) throws Exception {
return new Tuple2<>(value.f1, value.f2);
}
})
.name("group-by-longitude-latitude")
.sortByTimestamp(0);
// 利用 ProcessWindowFunction 计算每个窗口内经纬度范围内的对象数量
DataStream<Tuple3<Long, Double, Double>> resultStream = sortedStream
.keyBy(new KeySelector<Tuple3<Long, Double, Double>, Long>() {
@Override
public Long getKey(Tuple3<Long, Double, Double> value) throws Exception {
return value.f0;
}
})
.windowAll(TumblingEventTimeWindows.of(Time.seconds(10)))
.process(new CountObjectsInRegion());
// 将结果写入 PostgreSQL 中
Properties dbProps = new Properties();
dbProps.setProperty("url", "jdbc:postgresql://localhost:5432/dbname");
dbProps.setProperty("user", "username");
dbProps.setProperty("password", "password");
dbProps.setProperty("driver", "org.postgresql.Driver");
FlinkKafkaProducer<Tuple3<Long, Double, Double>> kafkaProducer = new FlinkKafkaProducer<>(
"output-topic",
new KeyedSerializationSchema<Tuple3<Long, Double, Double>>() {
@Override
public byte[] serializeKey(Tuple3<Long, Double, Double> element) {
return null;
}
@Override
public byte[] serializeValue(Tuple3<Long, Double, Double> element) {
return element.toString().getBytes();
}
@Override
public String getTargetTopic(Tuple3<Long, Double, Double> element) {
return null;
}
},
kafkaProps,
FlinkKafkaProducer.Semantic.EXACTLY_ONCE
);
resultStream
.addSink(new PostgreSQLSink<>(dbProps, "INSERT INTO table_name (timestamp, longitude, latitude, object_count) VALUES (?, ?, ?, ?)"));
env.execute("KafkaFlinkPostgreSQLExample");
}
}
```
上述代码中,`CountObjectsInRegion` 是一个继承 `ProcessWindowFunction` 的自定义类,用于计算每个窗口内经纬度范围内的对象数量。以下是 `CountObjectsInRegion` 类的一个可能的实现:
```java
import org.apache.flink.streaming.api.functions.windowing.ProcessWindowFunction;
import org.apache.flink.streaming.api.windowing.windows.TimeWindow;
import org.apache.flink.util.Collector;
public class CountObjectsInRegion extends ProcessWindowFunction<Tuple3<Long, Double, Double>, Tuple3<Long, Double, Double>, Long, TimeWindow> {
@Override
public void process(Long key, Context context, Iterable<Tuple3<Long, Double, Double>> elements, Collector<Tuple3<Long, Double, Double>> out) throws Exception {
double minLongitude = 116.0;
double maxLongitude = 117.0;
double minLatitude = 29.0;
double maxLatitude = 30.0;
int count = 0;
for (Tuple3<Long, Double, Double> element : elements) {
if (element.f1 >= minLongitude && element.f1 <= maxLongitude && element.f2 >= minLatitude && element.f2 <= maxLatitude) {
count++;
}
}
out.collect(new Tuple3<>(context.window().getEnd(), maxLongitude, maxLatitude, count));
}
}
```
上述代码中,`process` 方法中的 `elements` 参数是一个经过 KeyBy 和窗口操作后的迭代器,其中包含了所有经过该窗口的元素。`out` 参数是一个 `Collector` 对象,用于输出计算结果。
需要注意的是,上述代码中使用了 `org.apache.flink.streaming.connectors.postgres.PostgreSQLSink`,这是一个第三方库,需要手动添加到项目中。你可以通过 Maven 或 Gradle 管理依赖,具体请参考该库的文档。
相关推荐
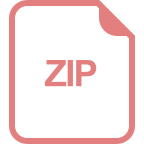
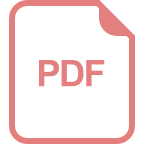














