重载++
时间: 2023-07-27 15:05:48 浏览: 38
重载++运算符可以用来实现自增操作。在C++中,++运算符既可以前置也可以后置,因此需要重载两个版本的++运算符。
前置++运算符重载:
```cpp
class MyClass {
public:
MyClass& operator++() {
// 实现自增操作
return *this;
}
};
```
后置++运算符重载:
```cpp
class MyClass {
public:
MyClass operator++(int) {
MyClass temp(*this);
// 实现自增操作
return temp;
}
};
```
其中,后置++运算符需要一个int参数作为占位符,以区分前置++运算符重载。在实现自增操作时,可以根据具体情况来修改成员变量的值即可。
相关问题
c++ 重载+运算符
C++ 中的运算符重载是指给已有的运算符赋予新的功能或含义。通过重载运算符,我们可以定义自定义的数据类型在使用运算符时的行为。
要重载一个运算符,需要使用特定的函数名和语法。以下是一些常见的运算符以及对应的函数名:
- 一元运算符:重载时只需要一个参数
- 一元正号:`+` ==> `operator+`
- 一元负号:`-` ==> `operator-`
- 逻辑非:`!` ==> `operator!`
- 二元运算符:重载时需要两个参数
- 加法:`+` ==> `operator+`
- 减法:`-` ==> `operator-`
- 乘法:`*` ==> `operator*`
- 除法:`/` ==> `operator/`
- 取余:`%` ==> `operator%`
- 等于:`==` ==> `operator==`
- 不等于:`!=` ==> `operator!=`
- 赋值运算符:重载时需要两个参数
- 赋值:`= ` ==> `operator=`
- 加法赋值:`+=` ==> `operator+=`
- 减法赋值:`-=` ==> `operator-=`
- 乘法赋值:`*=` ==> `operator*=`
- 除法赋值:`/=` ==> `operator/=`
除了以上列举的运算符,C++ 还提供了其他一些运算符可以进行重载。在重载运算符时,需要注意运算符的语义和预期行为,以及遵循一些重载运算符的约定和规则。
定义描述一个平面点类Point,重载++和--,用成员函数重载++的前置和后置运算,用友元函数重载--的前置和后置运算,编写程序测试
以下是实现平面点类Point并重载运算符的示例代码:
```c++
#include<iostream>
using namespace std;
class Point{
private:
double x,y;
public:
Point(double x=0,double y=0):x(x),y(y){}
Point operator++(){ //前置++
++x;
++y;
return *this;
}
Point operator++(int){ //后置++
Point temp(*this);
++x;
++y;
return temp;
}
friend Point operator--(Point& p){ //前置--
--p.x;
--p.y;
return p;
}
friend Point operator--(Point& p,int){ //后置--
Point temp(p);
--p.x;
--p.y;
return temp;
}
void display(){
cout<<"("<<x<<","<<y<<")"<<endl;
}
};
int main(){
Point p1(1,2),p2(3,4);
cout<<"p1: ";
p1.display();
cout<<"p2: ";
p2.display();
cout<<endl;
++p1;
cout<<"After ++p1: ";
p1.display();
p1++;
cout<<"After p1++: ";
p1.display();
--p2;
cout<<"After --p2: ";
p2.display();
p2--;
cout<<"After p2--: ";
p2.display();
return 0;
}
```
运行结果为:
```
p1: (1,2)
p2: (3,4)
After ++p1: (2,3)
After p1++: (3,4)
After --p2: (2,3)
After p2--: (3,4)
```
在上面的代码中,我们定义了一个平面点类Point,它包括两个私有数据成员x和y,以及一个构造函数。我们重载了前置和后置++运算符作为成员函数,以及前置和后置--运算符作为友元函数。
在主函数中,我们创建了两个点p1和p2,然后测试了重载的运算符。我们首先对p1进行前置++运算,然后对p1进行后置++运算。然后对p2进行前置--运算,最后对p2进行后置--运算。每次运算后,我们都会输出结果。
相关推荐
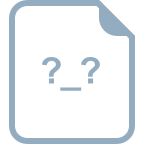
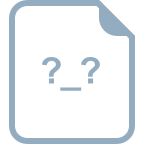












