在C#图像处理中,如何读取int一维数组的非零长度?
时间: 2024-02-16 11:03:36 浏览: 77
在C#中进行图像处理时,通常使用的是`Bitmap`类来读取图像数据。您可以使用`Bitmap.LockBits()`方法来锁定位图的指定区域,并获取该区域的内存地址,然后通过指针访问位图数据。
以下是一个示例代码,可以读取一个`Bitmap`对象中非零像素点的数量:
```csharp
using System.Drawing;
using System.Drawing.Imaging;
class Program
{
static void Main(string[] args)
{
Bitmap bitmap = new Bitmap("test.jpg");
Rectangle rect = new Rectangle(0, 0, bitmap.Width, bitmap.Height);
BitmapData bitmapData = bitmap.LockBits(rect, ImageLockMode.ReadOnly, PixelFormat.Format32bppArgb);
int[] pixels = new int[bitmapData.Width * bitmapData.Height];
System.Runtime.InteropServices.Marshal.Copy(bitmapData.Scan0, pixels, 0, pixels.Length);
int nonZeroCount = pixels.Count(p => p != 0);
bitmap.UnlockBits(bitmapData);
Console.WriteLine("The number of non-zero pixels is: {0}", nonZeroCount);
}
}
```
在上述代码中,我们首先使用`Bitmap.LockBits()`方法锁定了整张位图,然后使用`Marshal.Copy()`方法将位图数据复制到一个`int`类型的一维数组中。这个数组中的每个元素代表一个像素点的颜色值,如果该像素点是非零像素点,则其颜色值不为0。最后我们使用LINQ库中的`Count`方法来获取非零像素点的数量。
请注意,上述代码中没有对位图数据进行任何处理,因此这种方法只能用于读取灰度图像或二值图像中非零像素点的数量。如果您需要读取彩色图像中非零像素点的数量,则需要对位图数据进行更复杂的处理。
阅读全文
相关推荐
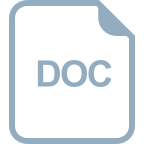
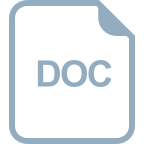
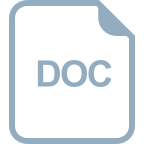
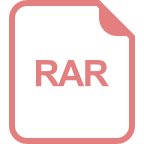
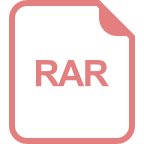
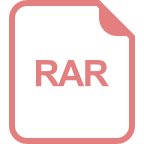
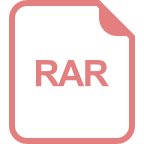
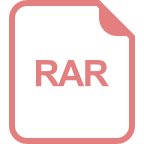
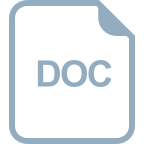
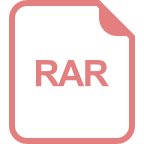
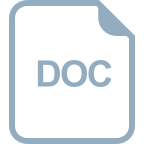
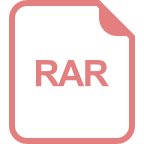
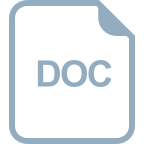
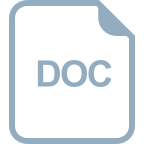
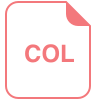
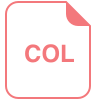
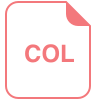
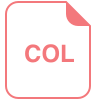
