ZipOutputStream closeEntry方法和close方法的区别
时间: 2024-04-28 20:27:07 浏览: 230
ZipOutputStream的closeEntry()方法和close()方法有不同的作用。
closeEntry()方法用于关闭当前已写入的ZipEntry,并将流定位到下一个条目的起始位置。当我们使用ZipOutputStream向zip文件中写入多个条目时,我们需要在写入一个条目完成后调用closeEntry()方法来关闭它,以便继续写入下一个条目。如果不调用closeEntry()方法,后续的数据可能会被写入到当前条目之后,导致压缩文件损坏。
close()方法用于关闭ZipOutputStream流,并释放与之相关的所有系统资源,包括关闭底层输出流。调用close()方法后,ZipOutputStream对象就不能再被使用了。因此,我们应该在所有条目都写入完成后,最后调用close()方法来关闭ZipOutputStream流。
综上所述,closeEntry()方法和close()方法都是ZipOutputStream中的重要方法,但是它们的作用不同,应该在不同的情况下使用。
相关问题
ZipOutputStream
ZipOutputStream is a class in Java that allows for the creation of ZIP files. It is part of the java.util.zip package and extends the DeflaterOutputStream class. ZipOutputStream can be used to create a new ZIP file or add entries to an existing ZIP file.
To create a new ZIP file using ZipOutputStream, first create a new instance of the class and pass it an OutputStream object. Then, use the putNextEntry method to create a new entry in the ZIP file and write data to it using the write method. When finished adding entries to the ZIP file, use the closeEntry method to close the current entry and finalize its contents. Lastly, use the finish method to finish writing the ZIP file and close the ZipOutputStream.
Example code for creating a new ZIP file using ZipOutputStream:
```
import java.io.*;
import java.util.zip.*;
public class CreateZipFile {
public static void main(String[] args) {
try {
FileOutputStream fos = new FileOutputStream("example.zip");
ZipOutputStream zos = new ZipOutputStream(fos);
// create a new entry in the zip file
ZipEntry entry = new ZipEntry("file.txt");
zos.putNextEntry(entry);
// write data to the entry
String data = "This is some example data.";
zos.write(data.getBytes());
// close the current entry
zos.closeEntry();
// finish writing the zip file
zos.finish();
zos.close();
System.out.println("Zip file created successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
zipoutputstream转inputstream另一种方法
除了使用字节数组作为中间缓存之外,还有一种更简单的方法来将 ZipOutputStream 转换为 InputStream,那就是使用 PipedOutputStream 和 PipedInputStream 组合起来使用。
1. 首先创建一个 PipedOutputStream 对象,用于将数据写入管道中。
2. 然后创建一个 PipedInputStream 对象,用于从管道中读取数据。
3. 将 PipedOutputStream 对象传递给 ZipOutputStream 的构造函数,这样 ZipOutputStream 就会将压缩数据写入 PipedOutputStream 中。
4. 将 PipedInputStream 对象传递给其他需要读取压缩数据的方法即可。
以下是示例代码:
```java
public static InputStream zipOutputStreamToInputStream() throws IOException {
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
ZipOutputStream zipOutputStream = new ZipOutputStream(byteArrayOutputStream);
zipOutputStream.putNextEntry(new ZipEntry("test.txt"));
zipOutputStream.write("Hello World".getBytes());
zipOutputStream.closeEntry();
zipOutputStream.close();
PipedOutputStream pipedOutputStream = new PipedOutputStream();
PipedInputStream pipedInputStream = new PipedInputStream(pipedOutputStream);
new Thread(() -> {
try {
pipedOutputStream.write(byteArrayOutputStream.toByteArray());
pipedOutputStream.flush();
pipedOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}).start();
return pipedInputStream;
}
```
在上面的示例代码中,我们将 ZipOutputStream 转换为 InputStream,最终返回的就是一个包含压缩数据的 InputStream 对象。
阅读全文
相关推荐
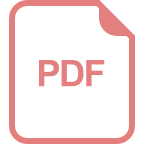
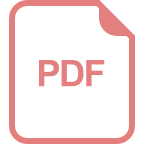
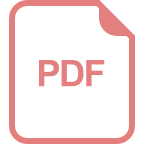













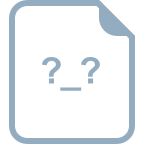