python if
时间: 2023-07-01 13:29:22 浏览: 88
In Python, `if` is a conditional statement that allows you to execute a block of code only if a certain condition is met. Here's the basic syntax of an `if` statement:
```python
if condition:
# code to execute if condition is True
```
The `condition` can be any expression that evaluates to a Boolean value (`True` or `False`). If the `condition` is `True`, the code block indented under the `if` statement will be executed. If the `condition` is `False`, the code block will be skipped and execution will continue with the next statement after the `if` block.
You can also use an `else` statement to specify a block of code to execute if the `condition` is `False`:
```python
if condition:
# code to execute if condition is True
else:
# code to execute if condition is False
```
Finally, you can use an `elif` statement (short for "else if") to specify additional conditions to check:
```python
if condition1:
# code to execute if condition1 is True
elif condition2:
# code to execute if condition2 is True and condition1 is False
else:
# code to execute if both condition1 and condition2 are False
```
Here's an example to help illustrate how `if` statements work:
```python
x = 5
if x > 0:
print("x is positive")
else:
print("x is non-positive")
```
In this example, the `condition` is `x > 0`. Since `x` is equal to `5`, which is greater than `0`, the code block under the `if` statement will be executed, and the output will be:
```
x is positive
```
I hope this helps! Let me know if you have any other questions.
阅读全文
相关推荐
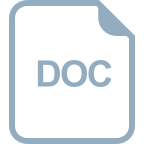
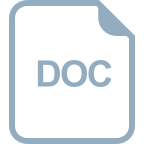
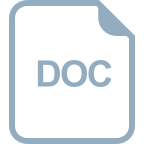
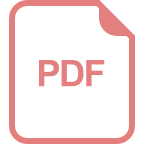
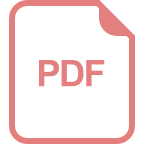
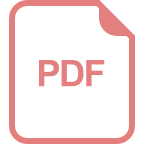
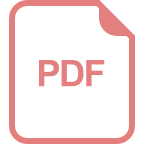
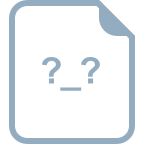
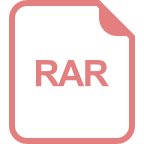
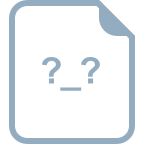
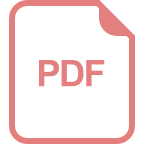
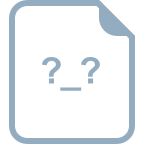
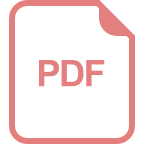
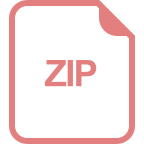
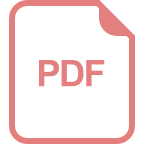
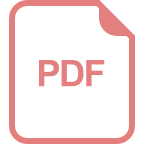
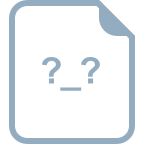
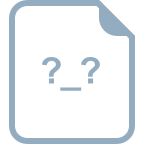