定义一个结构体变量,存放年,月,日,从键盘输入一个日期,计算并输出该日在该年中是第几天,注意该年是闰年的情况。
时间: 2023-10-01 19:09:15 浏览: 44
好的,可以使用以下代码实现:
```c
#include <stdio.h>
struct date {
int year;
int month;
int day;
};
int is_leap_year(int year) {
return ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0);
}
int day_of_year(struct date d) {
int days[] = {0, 31, 59, 90, 120, 151, 181, 212, 243, 273, 304, 334};
int day = days[d.month - 1] + d.day;
if (d.month > 2 && is_leap_year(d.year)) {
day++;
}
return day;
}
int main() {
struct date d;
printf("请输入一个日期(格式:年 月 日):");
scanf("%d %d %d", &d.year, &d.month, &d.day);
int day = day_of_year(d);
printf("%d年%d月%d日是该年的第%d天。\n", d.year, d.month, d.day, day);
return 0;
}
```
代码中,我们定义了一个 `date` 结构体来存放年月日信息,然后实现了两个函数:
- `is_leap_year()`:判断一个年份是否是闰年;
- `day_of_year()`:计算给定日期在给定年份中是第几天。
在 `day_of_year()` 函数中,我们使用一个数组 `days` 来存储每个月份的天数,然后根据输入日期的月份和日数计算出该日期在当年中的天数。如果该年是闰年,且输入日期在二月份之后,还需要将天数加 1。
最后在 `main()` 函数中,我们从键盘读入一个日期,调用 `day_of_year()` 函数计算天数,并输出结果。
相关推荐
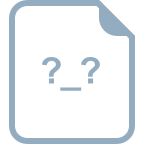
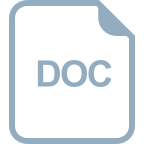
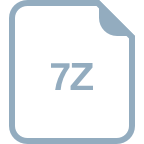














