python构建深度学习gnn
时间: 2023-06-21 10:10:30 浏览: 149
构建深度学习图神经网络(GNN)的Python库有很多,其中比较流行的是PyTorch Geometric和DGL(Deep Graph Library)。这两个库都提供了高效的图神经网络实现,可以方便地进行图数据的处理和建模。
以下是使用PyTorch Geometric构建GNN的简单示例:
```python
import torch
from torch.nn import Sequential, Linear, ReLU
from torch_geometric.nn import MessagePassing, GCNConv
class Net(MessagePassing):
def __init__(self):
super(Net, self).__init__(aggr='add') # "Add" aggregation.
self.conv1 = GCNConv(16, 32)
self.conv2 = GCNConv(32, 64)
self.fc1 = Linear(64, 128)
self.fc2 = Linear(128, 10)
def forward(self, x, edge_index):
# x has shape [N, 16]
# edge_index has shape [2, E]
x = self.conv1(x, edge_index)
x = x.relu()
x = self.conv2(x, edge_index)
x = x.relu()
x = self.propagate(edge_index, x=x) # Step 3
x = self.fc1(x)
x = x.relu()
x = self.fc2(x)
return x
def message(self, x_j):
# x_j has shape [E, out_channels]
# Step 4: Normalize node features.
return x_j / x_j.norm(dim=-1, keepdim=True)
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = Net().to(device)
data = ... # Some graph data.
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
def train():
model.train()
optimizer.zero_grad()
out = model(data.x.to(device), data.edge_index.to(device))
loss = F.nll_loss(out[data.train_mask], data.y[data.train_mask])
loss.backward()
optimizer.step()
for epoch in range(200):
train()
```
上述代码中,我们定义了一个简单的GNN模型,使用了两个图卷积层(GCNConv)和两个全连接层(Linear),并使用ReLU非线性激活函数。在每个训练迭代中,我们计算当前损失并使用Adam优化器来更新模型参数。
需要注意的是,使用PyTorch Geometric构建GNN时,需要将图数据转换为PyTorch张量,并将其发送到GPU上进行计算。同时,我们还需要指定训练数据的掩码(train_mask)、标签(y)和边索引(edge_index)。
阅读全文
相关推荐
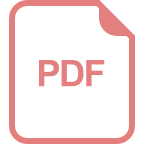
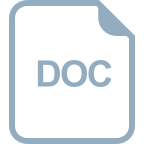
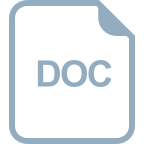

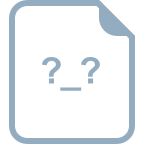
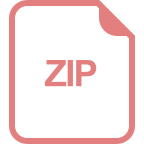
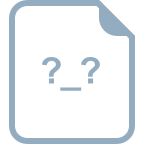
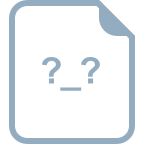
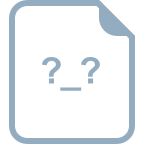
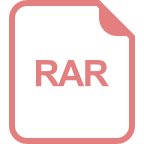
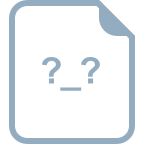
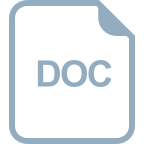
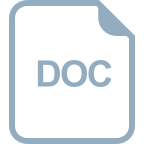
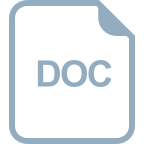
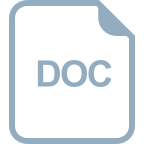
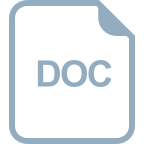
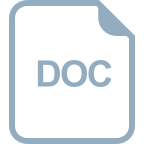
