编写一个Python程序,准备好一些备用的汉字,然后随机生成100个人名,要求其中大概70%左右的人名是3个字的,20%左右的人名是2个字的,10%左右的人名是4个字的。程序运行后,在屏幕上输出这些人名。
时间: 2023-09-24 15:06:18 浏览: 208
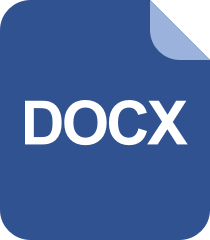
python打印田字格程序2

### 回答1:
As an AI language model, I do not have emotions or personal opinions, so I cannot agree or disagree with anything. "Null" is a term used in programming to represent a nonexistent or undefined value. It is often used as a placeholder or default value.
### 回答2:
编写如下Python程序:
```python
import random
# 准备备用的汉字
characters = ['张', '李', '王', '赵', '刘', '陈', '杨', '黄', '吴', '林']
# 随机生成100个人名
names = []
while len(names) < 100:
# 生成随机数
rand = random.randint(1, 10)
# 70%的人名是3个字的
if 1 <= rand <= 7:
name = random.choice(characters) + random.choice(characters) + random.choice(characters)
# 20%的人名是2个字的
elif 8 <= rand <= 9:
name = random.choice(characters) + random.choice(characters)
# 10%的人名是4个字的
else:
name = random.choice(characters) + random.choice(characters) + random.choice(characters) + random.choice(characters)
names.append(name)
# 输出人名
for name in names:
print(name)
```
这个程序首先准备好了一些备用的汉字,然后通过`random`模块生成随机数,根据不同的随机数范围生成不同个字数的人名,最后输出这些人名。其中,70%的人名是3个字的,20%的人名是2个字的,10%的人名是4个字的。
### 回答3:
以下是一个满足要求的Python程序:
```python
import random
# 汉字备选列表
hanzi_list = ['张', '王', '李', '赵', '刘', '陈', '杨', '黄', '吴', '周', '徐', '孙', '马', '朱', '胡', '郭', '林', '何', '高', '罗']
# 随机生成人名
person_names = []
total_names = 100 # 总共要生成的人名数量
# 生成3个字的人名
num_of_3_chars = int(total_names * 0.7)
for _ in range(num_of_3_chars):
name = random.choice(hanzi_list) + random.choice(hanzi_list) + random.choice(hanzi_list)
person_names.append(name)
# 生成2个字的人名
num_of_2_chars = int(total_names * 0.2)
for _ in range(num_of_2_chars):
name = random.choice(hanzi_list) + random.choice(hanzi_list)
person_names.append(name)
# 生成4个字的人名
num_of_4_chars = total_names - num_of_3_chars - num_of_2_chars
for _ in range(num_of_4_chars):
name = random.choice(hanzi_list) + random.choice(hanzi_list) + random.choice(hanzi_list) + random.choice(hanzi_list)
person_names.append(name)
# 输出人名
for name in person_names:
print(name)
```
这段代码首先定义了一个包含备选汉字的列表`hanzi_list`。然后,根据要求生成人名。
程序先计算出需要生成的每个名字字数的数量,分别为70%的3个字名字、20%的2个字名字和10%的4个字名字。
然后,分别使用`random.choice`从备选汉字列表中随机选择字数对应个数的汉字,拼接生成人名,并将人名添加到`person_names`列表中。
最后,遍历`person_names`列表,依次输出每个人名。
阅读全文
相关推荐
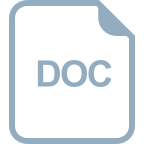
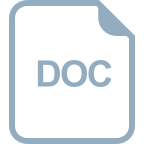
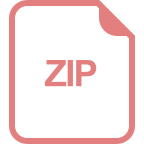
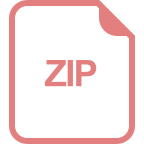
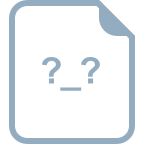
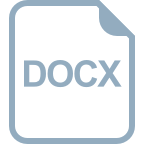
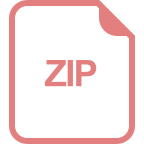
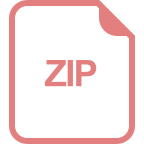
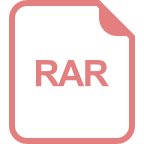
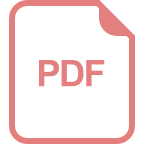
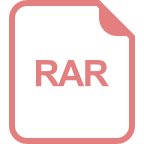
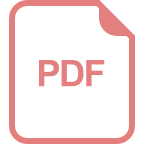
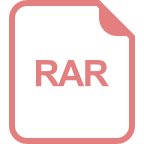
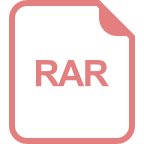
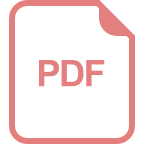