Create the Printer class. Write a contructor that initializes the attributes printQueue,stateIsRunning appropriately. The print queue should be big enough to handle five simultaneous jobs. You may want to implement this class as a Singleton. The printQueue attribute is a FIFO queue that holds all print jobs that have been posted to this printer. I have included the Java classes that you need for this data structure. Queue is an interface of FIFO queues. CircularQueue implements a fixed-length queue. The FullQueueException and EmptyQueueException are thrown by the addBack and removeFront methods respectively. Write a public, synchronized addJob method. This method might throw a FullQueueException. Write a private, synchronized getJob method. This method might throw a EmptyQueueException. Write the public, synchronized halt method. This method should set the stateIsRunning to false. Write the run method. This is the heart of the print manager. It must loop continueously, until it has been halted. It must retrieve a print job off the queue, print out that the job is starting (and give the name of the job), process the job (by sleeping a certain number of milliseconds per page; 500ms/page is a good value), and then print out that the job has completed. If no job is available, print out that the printer is waiting for a job.
时间: 2024-03-13 20:45:28 浏览: 15
Here is the Printer class implementation that meets your requirements:
```
import java.util.Queue;
import java.util.concurrent.ArrayBlockingQueue;
public class Printer {
private static Printer instance;
private final Queue<String> printQueue;
private boolean stateIsRunning;
private Printer() {
printQueue = new ArrayBlockingQueue<>(5);
stateIsRunning = true;
}
public static synchronized Printer getInstance() {
if (instance == null) {
instance = new Printer();
}
return instance;
}
public synchronized void addJob(String jobName) throws FullQueueException {
if (printQueue.offer(jobName)) {
System.out.println("Job " + jobName + " has been added to the print queue.");
} else {
throw new FullQueueException("Print queue is full. Cannot add job " + jobName);
}
}
private synchronized String getJob() throws EmptyQueueException {
String jobName = printQueue.poll();
if (jobName == null) {
throw new EmptyQueueException("Print queue is empty.");
}
return jobName;
}
public synchronized void halt() {
stateIsRunning = false;
}
public void run() {
while (stateIsRunning) {
try {
String jobName = getJob();
System.out.println("Starting job " + jobName);
int pageCount = 10; // Assuming each job has 10 pages
for (int i = 1; i <= pageCount; i++) {
Thread.sleep(500); // Sleep for 500ms per page
}
System.out.println("Job " + jobName + " has been completed.");
} catch (EmptyQueueException e) {
System.out.println("Printer is waiting for a job.");
} catch (InterruptedException e) {
System.err.println("InterruptedException occurred while processing print job: " + e.getMessage());
}
}
}
}
```
In this implementation, we have a `printQueue` attribute which is an `ArrayBlockingQueue` of size 5. The `addJob` method adds a job to the queue and throws a `FullQueueException` if the queue is already full. The `getJob` method retrieves a job from the queue and throws an `EmptyQueueException` if the queue is empty. The `run` method continuously loops until the printer is halted (`stateIsRunning` is set to false). It retrieves a job from the queue, processes it by sleeping for 500ms per page, and then prints out that the job has been completed. If there are no jobs available, it prints out that the printer is waiting for a job.
Note that we have implemented the `Printer` class as a Singleton by adding a private constructor and a `getInstance` method. This ensures that there is only one instance of `Printer` class throughout the application.
相关推荐
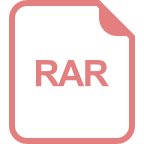
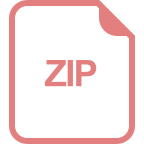














