import cv2 import socket import numpy as np # 创建socket连接 client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # 设置服务器地址和端口号 server_address = ('10.132.11.225', 8000) # 连接服务器 try: client_socket.connect(server_address) except socket.error as e: print("Failed to connect to server: {}".format(e)) exit() # 采集摄像头中的照片 cap = cv2.VideoCapture(0) # 检查摄像头是否打开成功 if not cap.isOpened(): print("Failed to open camera") exit() # 发送照片到服务器 while True: # 采集一帧照片 ret, frame = cap.read() # 检查照片采集是否成功 if not ret: print("Failed to capture frame") break # 将照片转换为JPEG格式,并压缩 _, jpeg = cv2.imencode('.jpg', frame, [int(cv2.IMWRITE_JPEG_QUALITY), 70]) # 判断数据大小是否符合要求 if len(jpeg) > 1024 * 1024: print("Data size too large") continue # 将JPEG数据转换为numpy数组,发送到服务器 data = np.array(jpeg).tobytes() # 发送数据到服务器 try: client_socket.sendall(data) # 接收确认信息 response = client_socket.recv(1024) if response != b'OK': print("Failed to receive response from server") break except socket.error as e: print("Failed to send data to server: {}".format(e)) break # 等待按下ESC键退出循环 if cv2.waitKey(1) == 27: break # 关闭socket连接和摄像头 client_socket.close() cap.release() cv2.destroyAllWindows()增加一个可视化窗口,增加使用按键确定照片
时间: 2024-02-14 10:16:26 浏览: 16
可以在while循环中添加如下代码来增加可视化窗口,并且使用按键确定照片:
```python
while True:
# 采集一帧照片
ret, frame = cap.read()
# 检查照片采集是否成功
if not ret:
print("Failed to capture frame")
break
# 在窗口中显示照片
cv2.imshow('frame', frame)
# 等待按下空格键拍摄照片
if cv2.waitKey(1) == ord(' '):
# 将照片转换为JPEG格式,并压缩
_, jpeg = cv2.imencode('.jpg', frame, [int(cv2.IMWRITE_JPEG_QUALITY), 70])
# 判断数据大小是否符合要求
if len(jpeg) > 1024 * 1024:
print("Data size too large")
continue
# 将JPEG数据转换为numpy数组,发送到服务器
data = np.array(jpeg).tobytes()
# 发送数据到服务器
try:
client_socket.sendall(data)
# 接收确认信息
response = client_socket.recv(1024)
if response != b'OK':
print("Failed to receive response from server")
break
except socket.error as e:
print("Failed to send data to server: {}".format(e))
break
# 等待按下ESC键退出循环
elif cv2.waitKey(1) == 27:
break
```
在每次采集一帧照片后,使用`cv2.imshow()`函数在窗口中显示照片。然后等待按下空格键,将当前帧照片转换为JPEG格式,并发送到服务器。如果按下ESC键,则退出循环。
相关推荐
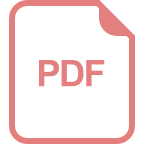
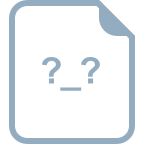










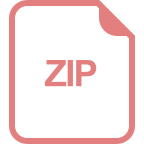
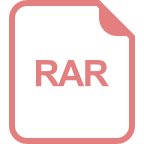
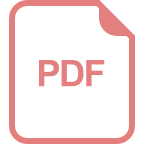
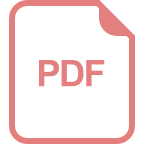
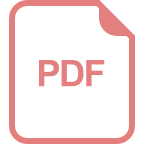
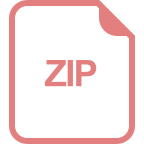