c语言使用尾插法建立一张带头结点的单链表L,并使L的长度大于10。将值为100的新结点*s插入到单链表L第4个结点位置
时间: 2024-05-15 09:17:43 浏览: 101
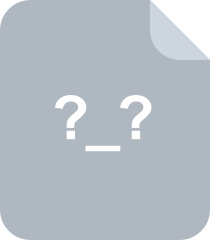
尾插法建立带头结点的单链表

你好!感谢你选择使用ChitGPT来进行对话。关于你的问题,可以这样实现:
首先,我们需要定义单链表的结构体和新节点的结构体:
```
typedef struct node {
int data;
struct node *next;
} Node;
typedef struct new_node {
int data;
struct node *next;
} NewNode;
```
然后,我们需要编写一个尾插法建立单链表的函数:
```
Node * createList(int n) {
Node *head, *p, *q;
int i;
head = (Node *)malloc(sizeof(Node));
head->next = NULL;
q = head;
for (i = 1; i <= n; i++) {
p = (Node *)malloc(sizeof(Node));
p->data = i * 10;
q->next = p;
q = p;
}
return head;
}
```
接着,我们需要在单链表的第四个位置插入一个值为100的新节点:
```
void insertNode(Node *head, NewNode *s, int position) {
Node *p, *q;
int n = 1;
p = head;
while (p && n < position) {
p = p->next;
n++;
}
if (!p || n > position) {
printf("插入位置无效\n");
return;
}
q = (Node *)malloc(sizeof(Node));
q->data = s->data;
q->next = p->next;
p->next = q;
}
```
最后,我们可以调用函数来实现题目所需的要求:
```
int main() {
Node *L = createList(11);
NewNode *s = (NewNode *)malloc(sizeof(NewNode));
s->data = 100;
insertNode(L, s, 4);
printf("单链表的数据为:");
Node *p = L->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return 0;
}
```
希望能对你有所帮助!
阅读全文
相关推荐

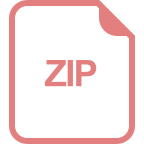

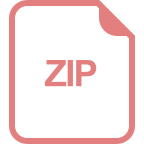
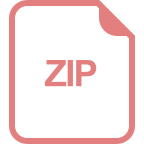
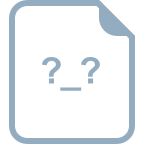
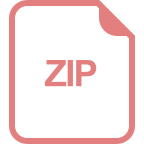
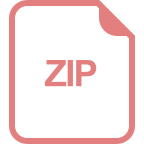
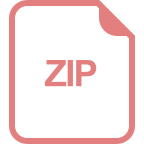
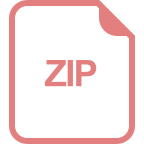
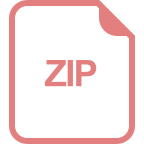
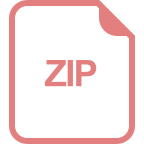
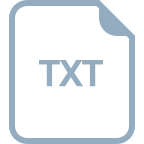