vue 指定单文件夹打包配置 demo
时间: 2023-09-10 07:06:56 浏览: 90
如果你要打包一个包含多个 Vue 单文件的文件夹,你可以通过配置 webpack 的 `entry` 选项来实现。假设你的 Vue 单文件都放在 `src/components` 文件夹下,那么可以将 `src/components` 文件夹下的所有 Vue 文件都打包成一个文件。
下面是一个简单的 webpack 配置示例:
```javascript
const path = require('path')
module.exports = {
entry: './src/components', // 入口文件为 src/components 文件夹
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'components.js'
},
module: {
rules: [
{
test: /\.vue$/,
loader: 'vue-loader'
}
]
}
}
```
这里的 `entry` 配置指向了 `src/components` 文件夹,webpack 会将该文件夹下的所有文件都打包成一个文件。同时,我们还使用了 `vue-loader` 来处理 Vue 单文件。最终打包后的文件会生成在 `dist` 文件夹下的 `components.js` 文件中。
相关问题
帮我写一个springboot vue 文件夹打包下载本地静态资源的代码实现
好的,以下是代码实现:
前端代码:
```vue
<template>
<div>
<button @click="downloadFile">下载文件</button>
</div>
</template>
<script>
export default {
methods: {
downloadFile() {
window.open("/statics.zip");
},
},
};
</script>
```
后端代码:
```java
package com.example.demo.controller;
import org.springframework.boot.configurationprocessor.json.JSONException;
import org.springframework.boot.configurationprocessor.json.JSONObject;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
@Controller
public class FileDownloadController {
@GetMapping("/download/statics")
@ResponseBody
public ResponseEntity<byte[]> downloadStaticResources() throws IOException {
FileSystemResource fileSystemResource =
new FileSystemResource(compressStaticResources());
byte[] fileBytes = new byte[(int) fileSystemResource.getFile().length()];
FileInputStream inputStream = new FileInputStream(fileSystemResource.getFile());
inputStream.read(fileBytes);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", "statics.zip");
headers.setContentLength(fileBytes.length);
return ResponseEntity.ok().headers(headers).body(fileBytes);
}
private String compressStaticResources() {
String tempZipPath = System.getProperty("java.io.tmpdir")+"/statics.zip";
try (ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(tempZipPath))) {
for (String staticsFolder : new String[]{"static", "public"}) {
try {
addFolderToZip(new ClassPathResource(staticsFolder).getFile(), staticsFolder, zos);
} catch (IOException e) {
e.printStackTrace();
}
}
return tempZipPath;
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return "";
}
private void addFolderToZip(File folder, String parentFolderName, ZipOutputStream zipOutputStream) throws IOException {
if (folder.isDirectory()) {
for (File file : folder.listFiles()) {
addFolderToZip(file, parentFolderName, zipOutputStream);
}
} else {
String name = folder.getName();
String path = folder.getPath().replace("\\", "/");
zipOutputStream.putNextEntry(new ZipEntry(path.substring(path.indexOf(parentFolderName), path.length())));
FileInputStream fileInputStream = new FileInputStream(folder);
byte[] bytes = new byte[1024];
int length;
while ((length = fileInputStream.read(bytes)) >= 0) {
zipOutputStream.write(bytes, 0, length);
}
fileInputStream.close();
}
}
}
```
这段代码使用了ZipOutputStream,将指定的静态资源文件夹进行了压缩,并通过@RepositoryRestController注解的Controller接口提供了下载静态资源文件的功能。
你可以在前端页面点击下载文件按钮,将会自动下载静态资源文件。同时,该代码也适用于任意SpringBoot+Vue项目。
使用vue.js创建移动端页面demo
Vue.js 是一款轻量级的前端框架,非常适合构建响应式的移动端页面。以下是一个简单的 Vue.js 创建移动端页面的步骤:
1. **安装 Vue**:
首先,你需要通过npm或yarn来安装Vue CLI(命令行工具)。打开终端,运行:
```bash
npm install -g @vue/cli
```
2. **创建项目**:
然后创建一个新的Vue项目:
```bash
vue create my-mobile-app
```
按照提示选择默认配置。
3. **进入项目目录**:
完成安装后,进入新创建的项目目录:
```bash
cd my-mobile-app
```
4. **准备移动端组件**:
在`src/components`下创建一个名为`MyMobileComponent.vue`的文件,添加基础的移动端布局:
```html
<template>
<div class="mobile-view">
<router-view></router-view>
</div>
</template>
<script>
export default {
name: 'MyMobileComponent',
}
</script>
<style scoped>
.mobile-view {
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
}
</style>
```
5. **路由设置**:
如果需要,可以配置路由以管理不同的页面。在`src/router/index.js`中添加相应的路由配置。
6. **开发并测试**:
使用`npm run serve`启动开发服务器,然后在浏览器的移动端查看效果,并使用Vue的响应式特性更新内容。
7. **运行应用**:
对于生产环境部署,记得运行`npm run build`打包项目,然后将dist文件夹的内容部署到服务器上。
阅读全文
相关推荐
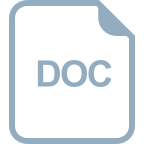
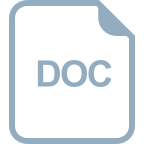
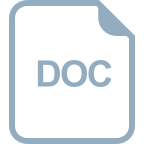
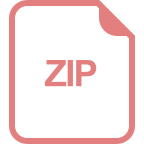
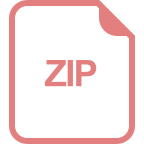
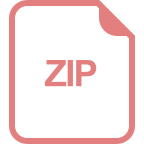
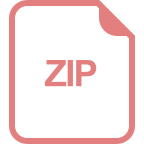
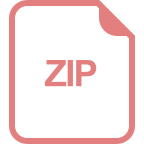
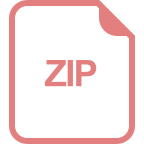
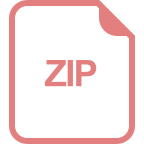
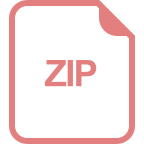
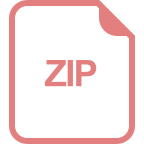
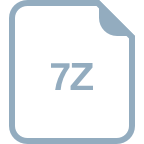
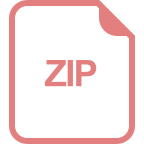
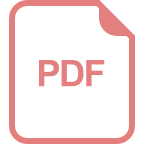
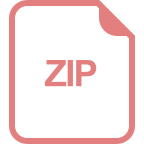
