用代码举例 java实现活体检测
时间: 2023-10-01 09:08:21 浏览: 211
活体检测是通过识别人脸是否为真实人脸,防止使用照片或者面具进行欺骗。下面是使用Java实现活体检测的示例代码:
首先需要引入OpenCV库,可以使用以下代码进行引入:
```java
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.core.MatOfRect;
import org.opencv.core.Point;
import org.opencv.core.Rect;
import org.opencv.core.Scalar;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.objdetect.CascadeClassifier;
```
然后使用以下代码进行初始化:
```java
// Load the native library.
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
// Load the haar cascade classifier for face detection
CascadeClassifier faceDetector = new CascadeClassifier("haarcascade_frontalface_alt.xml");
```
接下来是进行活体检测的代码:
```java
// Load the image file
Mat image = Imgcodecs.imread("test.jpg");
// Detect faces in the image
MatOfRect faceDetections = new MatOfRect();
faceDetector.detectMultiScale(image, faceDetections);
// For each detected face, perform liveness detection
for (Rect rect : faceDetections.toArray()) {
// Crop the face region
Mat face = image.submat(rect);
// Perform liveness detection on the face region
boolean isRealFace = performLivenessDetection(face);
// Draw a rectangle around the detected face
Imgproc.rectangle(image, new Point(rect.x, rect.y), new Point(rect.x + rect.width, rect.y + rect.height),
new Scalar(0, 255, 0));
// Write the result of the liveness detection on the image
if (isRealFace) {
Imgproc.putText(image, "Real Face", new Point(rect.x, rect.y - 5), Core.FONT_HERSHEY_SIMPLEX, 1,
new Scalar(0, 255, 0));
} else {
Imgproc.putText(image, "Fake Face", new Point(rect.x, rect.y - 5), Core.FONT_HERSHEY_SIMPLEX, 1,
new Scalar(0, 0, 255));
}
}
// Save the result image
Imgcodecs.imwrite("result.jpg", image);
```
其中,performLivenessDetection() 方法是用来进行活体检测的,具体实现可以根据具体需求选择不同的算法。
阅读全文
相关推荐





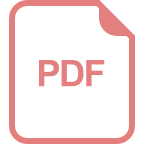

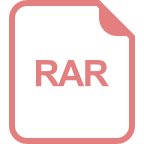

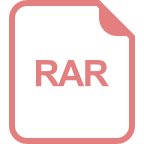



