帮我用pyqt5写(3) 车牌定位模块 车牌定位模块主要通过识别按钮,从视频流中拉取一帧图像送入车牌检测模型进行检测,并将检测的boundingbox进行裁剪,保存。 (4) 车牌识别模块 车牌识别模块接收车牌定位模块输入的车牌信息的图片,并采用百度飞桨的OCR识别获得车牌信息。
时间: 2024-02-13 13:00:43 浏览: 201
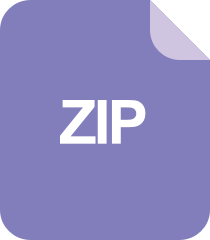
车牌识别,车牌检测,车辆定位。
以下是可能的PyQt5代码实现,包括车牌定位模块和车牌识别模块:
```python
import sys
import cv2
import numpy as np
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QMessageBox, QFileDialog
from PyQt5.QtGui import QImage, QPixmap
from paddleocr import PaddleOCR
class PlateDetectionWindow(QMainWindow):
def __init__(self):
super().__init__()
# 初始化界面
self.setWindowTitle('车牌定位模块')
self.setGeometry(100, 100, 800, 600)
# 设置界面控件
self.label = QLabel(self)
self.label.setGeometry(10, 10, 780, 480)
self.btn_detect = QPushButton('识别', self)
self.btn_detect.setGeometry(10, 500, 100, 50)
self.btn_detect.clicked.connect(self.detect_plate)
self.btn_save = QPushButton('保存', self)
self.btn_save.setGeometry(120, 500, 100, 50)
self.btn_save.clicked.connect(self.save_plate)
self.btn_quit = QPushButton('退出', self)
self.btn_quit.setGeometry(230, 500, 100, 50)
self.btn_quit.clicked.connect(self.close)
# 加载车牌检测模型
self.plate_detector = cv2.CascadeClassifier('haarcascade_russian_plate_number.xml')
# 初始化当前帧图像和检测到的车牌信息
self.current_frame = None
self.plate_box = None
def detect_plate(self):
# 从视频流中获取一帧图像
ret, frame = cap.read()
if not ret:
return
# 将图像转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 使用车牌检测模型检测车牌
plates = self.plate_detector.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(100, 50))
if len(plates) > 0:
# 取第一个检测到的车牌
self.plate_box = plates[0]
# 在原图上绘制车牌的bounding box
cv2.rectangle(frame, (self.plate_box[0], self.plate_box[1]),
(self.plate_box[0]+self.plate_box[2], self.plate_box[1]+self.plate_box[3]),
(0, 255, 0), 2)
# 裁剪车牌图像
plate_img = frame[self.plate_box[1]:self.plate_box[1]+self.plate_box[3],
self.plate_box[0]:self.plate_box[0]+self.plate_box[2]]
# 将裁剪后的车牌图像显示在界面上
self.current_frame = plate_img.copy()
else:
self.plate_box = None
self.current_frame = None
# 将原图转换为QImage格式并显示在界面上
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
h, w, ch = frame.shape
qimg = QImage(frame.data, w, h, ch*w, QImage.Format_RGB888)
pixmap = QPixmap.fromImage(qimg)
self.label.setPixmap(pixmap)
def save_plate(self):
if self.current_frame is not None and self.plate_box is not None:
# 弹出文件保存对话框,选择保存路径和文件名
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_name, _ = QFileDialog.getSaveFileName(self, "保存车牌图像", "", "JPEG Files (*.jpg);;All Files (*)", options=options)
if file_name:
# 保存车牌图像到指定路径
cv2.imwrite(file_name, self.current_frame)
QMessageBox.information(self, '提示', '车牌图像保存成功!')
class PlateRecognitionWindow(QMainWindow):
def __init__(self):
super().__init__()
# 初始化界面
self.setWindowTitle('车牌识别模块')
self.setGeometry(100, 100, 800, 600)
# 设置界面控件
self.label = QLabel(self)
self.label.setGeometry(10, 10, 780, 480)
self.btn_recognize = QPushButton('识别', self)
self.btn_recognize.setGeometry(10, 500, 100, 50)
self.btn_recognize.clicked.connect(self.recognize_plate)
self.btn_quit = QPushButton('退出', self)
self.btn_quit.setGeometry(120, 500, 100, 50)
self.btn_quit.clicked.connect(self.close)
# 加载OCR模型
self.ocr = PaddleOCR()
# 初始化当前车牌图像
self.current_plate = None
def recognize_plate(self):
if PlateDetectionWindow.current_frame is not None and PlateDetectionWindow.plate_box is not None:
# 将车牌图像转换为灰度图像
gray = cv2.cvtColor(PlateDetectionWindow.current_frame, cv2.COLOR_BGR2GRAY)
# 使用OCR模型识别车牌号码
result = self.ocr.ocr(gray, det=False)
plate_number = ''.join([r[1][0] for r in result])
# 在车牌图像上绘制识别结果
img = PlateDetectionWindow.current_frame.copy()
cv2.putText(img, plate_number, (10, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
# 将车牌图像转换为QImage格式并显示在界面上
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
h, w, ch = img.shape
qimg = QImage(img.data, w, h, ch*w, QImage.Format_RGB888)
pixmap = QPixmap.fromImage(qimg)
self.label.setPixmap(pixmap)
# 保存识别结果到文件
with open('plate_number.txt', 'a') as f:
f.write(f'{plate_number}\n')
f.flush()
else:
QMessageBox.warning(self, '提示', '请先进行车牌定位!')
if __name__ == '__main__':
# 初始化视频流
cap = cv2.VideoCapture(0)
# 初始化车牌定位模块和车牌识别模块
app = QApplication(sys.argv)
detection_window = PlateDetectionWindow()
recognition_window = PlateRecognitionWindow()
# 显示界面
detection_window.show()
recognition_window.show()
# 运行事件循环
sys.exit(app.exec_())
# 释放视频流
cap.release()
```
这段代码使用PyQt5实现了车牌定位模块和车牌识别模块。车牌定位模块从视频流中获取一帧图像,使用Haar级联分类器检测车牌,并将检测到的车牌图像显示在界面上。用户可以选择保存车牌图像。车牌识别模块接收车牌定位模块传输的车牌图像,使用百度飞桨的OCR模型识别车牌号码,并将识别结果显示在界面上。识别结果同时保存到文件中。用户可以在任意时刻启动车牌识别模块,但必须在车牌定位模块中获取到车牌图像后才能进行识别。
阅读全文
相关推荐
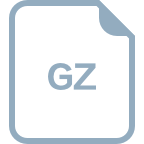
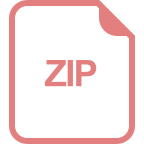
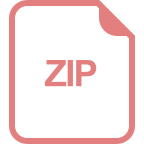
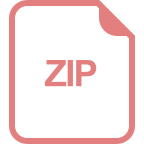
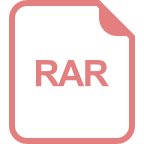
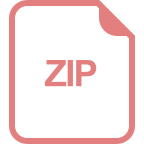
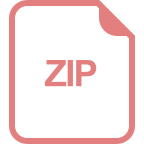
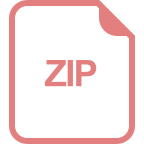
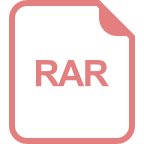
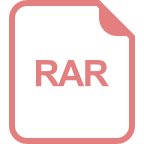
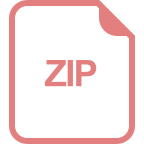
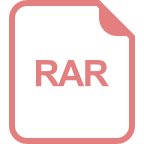
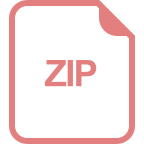
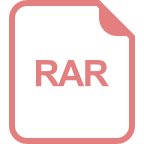
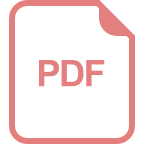
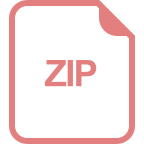
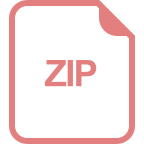