python xml child dict
时间: 2023-05-20 08:02:48 浏览: 197
可以使用xmltodict库将XML转换为Python字典,然后使用字典的子元素来访问XML的子元素。以下是一个示例代码:
import xmltodict
xml_string = '<root><person><name>John</name><age>30</age></person></root>'
xml_dict = xmltodict.parse(xml_string)
person_dict = xml_dict['root']['person']
name = person_dict['name']
age = person_dict['age']
print(name, age)
输出结果为:John 30
相关问题
Python xml转json
可以使用 Python 内置的 `xml.etree.ElementTree` 模块和 `json` 模块来实现 xml 转换为 json。
下面是一个简单的示例代码:
``` python
import xml.etree.ElementTree as ET
import json
# 读取 xml 文件
tree = ET.parse('sample.xml')
root = tree.getroot()
# 将 xml 转换为字典
xml_dict = {}
for child in root:
xml_dict[child.tag] = child.text
# 将字典转换为 json
json_data = json.dumps(xml_dict)
print(json_data)
```
这个示例代码假设 xml 文件的结构类似于:
``` xml
<root>
<name>John</name>
<age>30</age>
<gender>Male</gender>
</root>
```
如果 xml 文件的结构更加复杂,那么需要针对具体的情况做出相应的修改。
python解析xml文件转换成json
Python中有多种库可以用来解析XML文件并将其转换为JSON格式,其中最常用的是`xml.etree.ElementTree`库和`dicttojson`或者`python-dotenv`库结合`json`库。
1. 使用`xml.etree.ElementTree`库:
```python
import json
import xml.etree.ElementTree as ET
def xml_to_dict(element):
if len(element) == 0:
return element.text
result = {}
for child in element:
child_data = xml_to_dict(child)
if child.tag in result:
if type(result[child.tag]) is list:
result[child.tag].append(child_data)
else:
result[child.tag] = [result[child.tag], child_data]
else:
result[child.tag] = child_data
return result
def xml_to_json(xml_file_path, json_file_path):
tree = ET.parse(xml_file_path)
root = tree.getroot()
data = xml_to_dict(root)
with open(json_file_path, 'w') as f:
json.dump(data, f, indent=4)
# 示例
xml_to_json('your_xml_file.xml', 'output.json')
```
2. 使用`dicttojson`库:
```python
from dicttojson import dicttojson
def parse_xml_to_json(xml_content):
# 首先需要将XML字符串转换为ElementTree对象
from xml.dom.minidom import parseString
dom = parseString(xml_content)
root = dom.documentElement
return dicttojson(root)
# 示例
with open('your_xml_file.xml', 'r') as f:
xml_string = f.read()
json_output = parse_xml_to_json(xml_string)
print(json.dumps(json_output, indent=4))
```
注意替换`'your_xml_file.xml'`为你的实际XML文件路径。执行后,你会得到一个JSON格式的数据结构,然后你可以选择保存到文件或者直接操作。
阅读全文
相关推荐


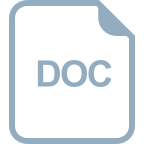











