torch::Tensor
时间: 2023-03-30 14:03:09 浏览: 76
可以用来做什么?
作为一个 AI 语言模型,我可以告诉你,torch::Tensor 是一个多维数组,可以用于高效地进行数值计算和深度学习模型的构建和训练。它支持各种数学运算和操作,如加、减、乘、除、矩阵乘法、卷积等,同时也支持 GPU 加速,能够大大提高计算速度。
相关问题
torch::Tensor to caffe2::Tensor
To convert a `torch::Tensor` to a `caffe2::Tensor`, you can use the `caffe2::TensorCPU` class, which provides a constructor that takes a pointer to data and a vector of dimensions. Here's an example:
```
#include <caffe2/core/tensor.h>
#include <torch/torch.h>
int main() {
// Create a torch::Tensor
torch::Tensor t = torch::ones({2, 3});
// Convert to a caffe2::Tensor
caffe2::TensorCPU c2_tensor((float*)t.data_ptr(), {2, 3});
return 0;
}
```
In this example, we create a `torch::Tensor` with dimensions 2x3 and fill it with ones. Then, we pass a pointer to the data and the dimensions to the `caffe2::TensorCPU` constructor to create a `caffe2::Tensor`. Note that we cast the data pointer to a `float*` because `caffe2::TensorCPU` expects a pointer to float data.
torch::Tensor 转为 caffe2::Tensor
可以使用以下代码将 torch::Tensor 转换为 caffe2::Tensor:
```c++
#include <caffe2/core/tensor.h>
#include <torch/script.h>
caffe2::Tensor convert_torch_to_caffe2(const torch::Tensor& input_tensor) {
// 获取张量的形状和数据类型
auto shape = input_tensor.sizes();
caffe2::TypeMeta data_type;
if (input_tensor.dtype() == torch::kFloat) {
data_type = caffe2::TypeMeta::Make<float>();
} else if (input_tensor.dtype() == torch::kInt) {
data_type = caffe2::TypeMeta::Make<int>();
} else {
throw std::runtime_error("Unsupported data type");
}
// 创建 caffe2::Tensor
caffe2::Tensor output_tensor(data_type, caffe2::DeviceType::CPU);
output_tensor.Resize(shape);
// 将数据从 torch::Tensor 复制到 caffe2::Tensor
if (input_tensor.is_contiguous()) {
std::memcpy(output_tensor.mutable_data(), input_tensor.data_ptr(), input_tensor.nbytes());
} else {
auto input_tensor_contiguous = input_tensor.contiguous();
std::memcpy(output_tensor.mutable_data(), input_tensor_contiguous.data_ptr(), input_tensor_contiguous.nbytes());
}
return output_tensor;
}
```
这个函数将 `torch::Tensor` 转换为 `caffe2::Tensor` 并返回。注意,这个函数只支持 `float` 和 `int` 数据类型。如果需要支持其他数据类型,需要相应地修改代码。
相关推荐
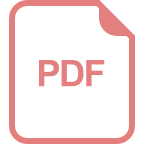
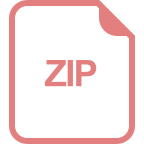
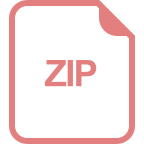












