c++编写fun函数删除字符串中的数字字符
时间: 2023-02-28 14:47:33 浏览: 138
可以使用 C 语言中的字符处理函数和循环语句来实现删除字符串中的数字字符。
以下是一个简单的实现方法:
```
#include <ctype.h>
#include <stdio.h>
#include <string.h>
void fun(char* str) {
int i, j = 0;
int len = strlen(str);
for (i = 0; i < len; i++) {
if (!isdigit(str[i])) {
str[j++] = str[i];
}
}
str[j] = '\0';
}
int main() {
char str[] = "Hello123World456";
fun(str);
printf("%s\n", str);
return 0;
}
```
输出:
```
HelloWorld
```
相关问题
用C++编写函数fun从字符串中删除指定的字符。同一字母的大、小写按不同字符处理。
以下是一个用C++实现的函数fun,可以从字符串中删除指定的字符,并对同一字母的大、小写按不同字符处理:
```c++
#include <iostream>
#include <string>
using namespace std;
string fun(string s, char c) {
string result = "";
for (int i = 0; i < s.length(); i++) {
if (s[i] != c && s[i] != toupper(c) && s[i] != tolower(c)) {
result += s[i];
}
}
return result;
}
int main() {
string s = "Hello World";
char c = 'o';
string result = fun(s, c);
cout << result << endl; // 输出 "Hell Wrld"
return 0;
}
```
函数fun的参数为字符串s和字符c,返回值为删除指定字符后的新字符串。在函数内部,我们使用for循环遍历字符串s中的每个字符,如果字符不等于指定字符c,也不等于其大、小写形式,就将其添加到结果字符串result中。最后返回result即可。
需要注意的是,在判断同一字母的大、小写形式时,需要用到C++内置函数toupper和tolower,分别将字符转换为大写和小写形式。这样才能确保同一字母的大、小写形式被当作不同字符处理。
希望对你有所帮助!
编写一个函数fun利用函数删除字符串中的数字字符利用c++实现
好的,下面是一个利用函数删除字符串中数字字符的C++函数实现:
```c++
#include <iostream>
#include <string>
using namespace std;
bool isDigit(char c) {
return c >= '0' && c <= '9';
}
string fun(string s, bool (*isDigit)(char)) {
string res = "";
for (char c : s) {
if (!isDigit(c)) {
res += c;
}
}
return res;
}
int main() {
string s = "abc123def456";
string res = fun(s, isDigit);
cout << res << endl; // 输出"abcdef"
return 0;
}
```
该函数使用一个循环遍历输入字符串中的每个字符,如果该字符不是数字字符,则将其添加到结果字符串中。函数的第二个参数是一个指向函数的指针,该函数用于判断输入字符是否为数字字符。在主函数中,我们演示了如何使用该函数来删除字符串中的数字字符,并将结果输出到控制台。
阅读全文
相关推荐
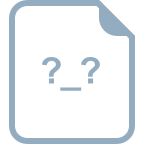












