用paddle分别实现正交位置编码和相对位置编码
时间: 2023-08-17 12:05:24 浏览: 47
好的,下面我来回答你的问题。
首先,正交位置编码和相对位置编码是Transformer模型中用于处理序列信息的两种编码方式。
正交位置编码是根据位置信息计算出一个固定的编码向量,不考虑序列中其他位置之间的关系。
相对位置编码则是考虑了序列中不同位置之间的相对关系,计算出不同位置之间的编码向量,可以更好地表达序列信息。
在PaddlePaddle中,我们可以使用PaddleNLP库中的PositionalEmbedding模块来实现正交位置编码和相对位置编码。下面是具体示例代码:
```python
import paddle
import paddle.nn as nn
import paddle.nn.functional as F
from paddlenlp.layers import PositionalEmbedding
# 正交位置编码
class OrthogonalPositionalEncoding(nn.Layer):
def __init__(self, d_model, max_len=512):
super().__init__()
self.pos_embedding = PositionalEmbedding(d_model, max_len)
def forward(self, x):
x = x + self.pos_embedding(paddle.arange(x.shape[1], dtype='float32'))
return x
# 相对位置编码
class RelativePositionalEncoding(nn.Layer):
def __init__(self, d_model, max_len=512):
super().__init__()
self.pos_embedding = nn.Embedding(max_len, d_model)
self.query_proj = nn.Linear(d_model, d_model, bias_attr=False)
self.key_proj = nn.Linear(d_model, d_model, bias_attr=False)
def forward(self, x):
pos = paddle.arange(x.shape[1], dtype='int64')
pos = paddle.unsqueeze(pos, axis=0)
pos_embedding = self.pos_embedding(pos)
query = self.query_proj(x)
key = self.key_proj(pos_embedding)
attn_weights = paddle.matmul(query, key.transpose([0, 2, 1])) / paddle.sqrt(paddle.to_tensor(query.shape[-1], dtype='float32'))
attn_weights = F.softmax(attn_weights, axis=-1)
out = paddle.matmul(attn_weights, pos_embedding)
return x + out
```
以上就是用Paddle实现正交位置编码和相对位置编码的示例代码,希望能够对你有所帮助。
相关推荐
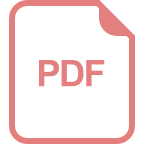
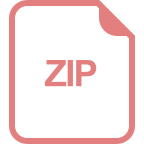
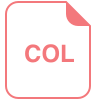













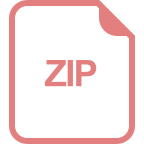