基于Python的拼图游戏,用户能够自行导入图片,图片分解为15张分布在4*4的棋盘上
时间: 2024-03-10 15:49:17 浏览: 153
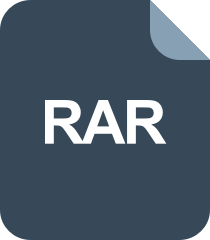
使用python实现的拼图游戏
好的,下面是基于Python的自定义图片拼图游戏的示例代码:
```python
import pygame
import os
import random
# 游戏窗口大小
WIDTH = 640
HEIGHT = 640
# 拼图块大小
TILE_SIZE = int(WIDTH / 4)
# 加载图片并切分拼图块
def load_image(file_name):
file_path = os.path.join('images', file_name)
return pygame.image.load(file_path)
def create_tiles(image):
tiles = []
for i in range(4):
for j in range(4):
rect = pygame.Rect(j*TILE_SIZE, i*TILE_SIZE, TILE_SIZE, TILE_SIZE)
tile = image.subsurface(rect)
tiles.append(tile)
return tiles
# 获取鼠标点击的拼图块编号
def get_tile_index(pos):
x, y = pos
row = y // TILE_SIZE
col = x // TILE_SIZE
return row * 4 + col
# 获取拼图块的位置
def get_tile_pos(index):
row = index // 4
col = index % 4
x = col * TILE_SIZE
y = row * TILE_SIZE
return (x, y)
# 移动拼图块
def move_tile(index1, index2, tiles):
tiles[index1], tiles[index2] = tiles[index2], tiles[index1]
# 交换拼图块
def swap_tiles(index1, index2, tiles):
move_tile(index1, index2, tiles)
# 处理鼠标点击事件
def handle_mouse_click(pos, tiles):
index = get_tile_index(pos)
blank_index = get_tile_index(get_tile_pos(len(tiles)-1))
if index == blank_index:
return
if index % 4 > 0 and index - 1 == blank_index:
swap_tiles(index, blank_index, tiles)
elif index % 4 < 3 and index + 1 == blank_index:
swap_tiles(index, blank_index, tiles)
elif index - 4 == blank_index:
swap_tiles(index, blank_index, tiles)
elif index + 4 == blank_index:
swap_tiles(index, blank_index, tiles)
# 主函数
def main():
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('拼图游戏')
# 加载图片并切分拼图块
image_file = input('请输入图片名称:')
image = load_image(image_file)
tiles = create_tiles(image)
# 随机打乱拼图块
random.shuffle(tiles)
# 启动主循环
clock = pygame.time.Clock()
game_over = False
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.MOUSEBUTTONDOWN:
handle_mouse_click(event.pos, tiles)
# 绘制拼图块
screen.fill((255, 255, 255))
for i, tile in enumerate(tiles):
pos = get_tile_pos(i)
screen.blit(tile, pos)
pygame.display.flip()
# 检查是否已经完成拼图
if tiles == sorted(tiles, key=lambda x: x.get_at((0, 0))):
print('恭喜你完成了拼图!')
break
clock.tick(60)
# 关闭Pygame
pygame.quit()
if __name__ == '__main__':
main()
```
在运行程序时,会提示用户输入图片名称,用户可以输入任意图片的名称,程序会在`images`文件夹下查找该图片,并将其切分成15个拼图块。然后,程序会随机打乱拼图块的位置,并启动主循环,等待用户操作拼图。用户点击拼图块时,程序会根据鼠标点击的位置计算出拼图块的编号,并根据编号计算出空白拼图块的位置,然后判断鼠标点击的拼图块是否可以移动,并在可以移动时交换拼图块的位置。每次交换拼图块后,程序会检查拼图是否已经完成,如果完成了拼图,则退出主循环并输出恭喜信息。
希望这个示例代码可以帮助你开发出自己的Python拼图游戏!
阅读全文
相关推荐
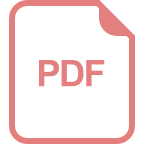
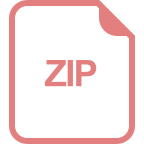
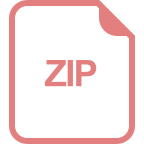
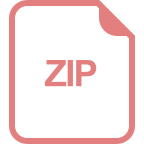
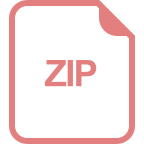
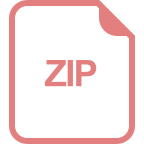
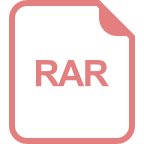
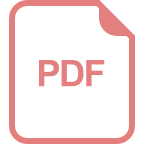
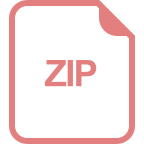
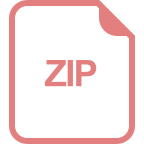
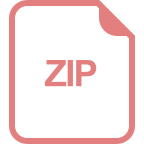