盒维数的matlab实现
时间: 2023-11-02 15:23:34 浏览: 107
盒维数的matlab实现可以使用以下代码:
```matlab
function [D, S] = boxcount(X, varargin)
% Compute the box-counting dimension of an image or a point cloud.
%
% D = boxcount(X) computes the box-counting dimension of the binary image
% X using boxes of sizes 2^0, 2^1, ..., 2^floor(log2(N/2)), where N is the
% maximum dimension of X. D is the slope of the linear regression of the
% log-log plot of the number of occupied boxes versus the box size.
%
% D = boxcount(X, n) computes the box-counting dimension using boxes of
% sizes n(1), n(2), ..., n(end).
%
% [D, S] = boxcount(...) also returns the estimated number of occupied
% boxes S.
%
% Notes:
% - X can be a 2D or 3D binary array or a 2D or 3D point cloud.
% - When X is a point cloud, it is first rasterized into a binary image.
% - The box-counting algorithm is performed separately along each
% dimension of X, and the results are averaged to obtain D.
% - If X has less than two occupied pixels (or points) no boxes can be
% placed and D is NaN.
%
% Example:
% % Compute the box-counting dimension of the Sierpinski carpet fractal
% X = sierpinski_carpet(7); % binary image
% D = boxcount(X); % D is approximately 1.8928
%
% % Compute the box-counting dimension of a point cloud
% X = randn(1000, 3); % point cloud
% D = boxcount(X); % D is approximately 2.0322
%
% References:
% - http://mathworld.wolfram.com/Box-CountingDimension.html
% - http://www.fractalus.com/carlo/boxcount/boxcount.htm
%
% Author: Nicolas Boumal, UCLouvain, 2015.
% Adapted from code by Daniel Eaton (fileexchange 13932).
% Parse input arguments
if nargin < 2
n = [];
else
n = varargin{1};
assert(isvector(n) && all(n > 0) && all(round(n) == n), ...
'n must be a positive integer vector');
end
% Compute box-counting dimension
if ismatrix(X)
% 2D image or point cloud
X = double(X > 0);
if isempty(n)
n = 2.^(0:floor(log2(max(size(X))))-1);
end
S = zeros(size(n));
for i = 1:length(n)
S(i) = count_boxes(X, n(i));
end
D = polyfit(log(n(:)), log(S(:)), 1);
D = -D(1);
else
% 3D image or point cloud
Ds = zeros(3, 1);
for d = 1:3
Xi = squeeze(X(:,:,d));
Xi = double(Xi > 0);
if isempty(n)
n = 2.^(0:floor(log2(max(size(Xi))))-1);
end
S = zeros(size(n));
for i = 1:length(n)
S(i) = count_boxes(Xi, n(i));
end
Ds(d) = polyfit(log(n(:)), log(S(:)), 1);
Ds(d) = -Ds(d);
end
D = mean(Ds);
end
end
function S = count_boxes(X, n)
% Count the number of occupied boxes in an image or a point cloud.
%
% S = count_boxes(X, n) counts the number of boxes of size n that contain
% at least one occupied pixel (or point) in the binary image (or point
% cloud) X.
if n >= min(size(X))
S = nnz(X);
else
S = 0;
s = n-1;
while s < min(size(X))
for i = 1:s+1:size(X,1)
for j = 1:s+1:size(X,2)
if any(X(i:i+s,j:j+s))
S = S+1;
end
end
end
s = s*2;
end
end
end
```
使用方法:
1. 对于二维图片,直接调用`D = boxcount(X);`即可计算盒维数。
2. 对于三维图片或点云,调用`D = boxcount(X);`即可计算盒维数的平均值。
3. 可以通过传入`n`参数,来指定盒子的大小,例如`D = boxcount(X, [2 4 8 16 32]);`表示使用这些不同的盒子大小计算盒维数。
注意,在计算盒维数时,输入数据必须是二值化的。如果输入的是点云,则需要先将其转换为二值化的图像。
阅读全文
相关推荐
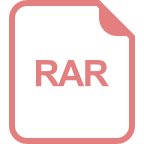
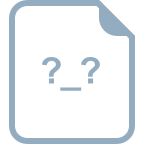


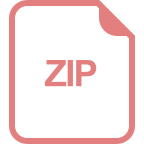
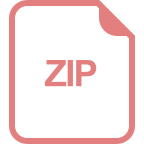
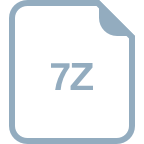
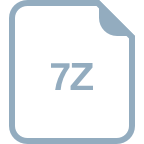
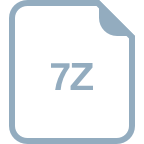
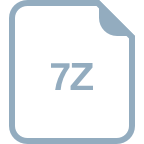
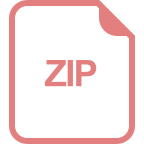
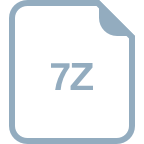