import tensorflow.compat.v1 as tf tf.disable_v2_behavior() from PIL import Image import matplotlib.pyplot as plt import input_data import model import numpy as np import xlsxwriter #设置线程数 num_threads = 4 def evaluate_one_image(): workbook = xlsxwriter.Workbook('formatting.xlsx') worksheet = workbook.add_worksheet('My Worksheet') with tf.Graph().as_default(): BATCH_SIZE = 1 N_CLASSES = 4 image = tf.cast(image_array, tf.float32) image = tf.image.per_image_standardization(image) image = tf.reshape(image, [1, 208, 208, 3]) logit = model.cnn_inference(image, BATCH_SIZE, N_CLASSES) logit = tf.nn.softmax(logit) x = tf.placeholder(tf.float32, shape=[208, 208, 3]) logs_train_dir = 'log/' saver = tf.train.Saver() with tf.Session() as sess: print("从指定路径中加载模型...") ckpt = tf.train.get_checkpoint_state(logs_train_dir) if ckpt and ckpt.model_checkpoint_path: global_step = ckpt.model_checkpoint_path.split('/')[-1].split('-')[-1] saver.restore(sess, ckpt.model_checkpoint_path) print('模型加载成功, 训练的步数为: %s' % global_step) else: print('模型加载失败,checkpoint文件没找到!') prediction = sess.run(logit, feed_dict={x: image_array}) max_index = np.argmax(prediction) workbook.close() def evaluate_images(test_img): coord = tf.train.Coordinator() threads = tf.train.start_queue_runners(coord=coord) for index,img in enumerate(test_img): image = Image.open(img) image = image.resize([208, 208]) image_array = np.array(image) tf.compat.v1.threading.Thread(target=evaluate_one_image, args=(image_array, index)).start() # 请求停止所有线程 coord.request_stop() # 等待所有线程完成 coord.join(threads) if __name__ == '__main__': # 调用方法,开始测试 test_dir = 'data/test/' import glob import xlwt test_img = glob.glob(test_dir + '*.jpg') evaluate_images(test_img)
时间: 2024-04-08 13:32:51 浏览: 124
这段代码是一个图像分类的程序。它使用了TensorFlow框架和PIL库进行图像处理,以及xlsxwriter库进行Excel文件的写入。程序主要包括两个函数:evaluate_one_image和evaluate_images。evaluate_one_image函数用于对单张图像进行分类,而evaluate_images函数用于对多张图像进行分类。
在evaluate_one_image函数中,首先将图像进行预处理,然后加载训练好的模型并进行预测,最后将预测结果写入Excel文件。
在evaluate_images函数中,首先创建一个线程协调器和多个线程来处理多张图像,然后调用evaluate_one_image函数对每张图像进行分类。
在主程序中,首先指定测试图像的路径,然后调用evaluate_images函数进行图像分类。
请注意,这段代码只是一个框架,其中的一些变量和函数需要根据实际情况进行修改和补充。
相关问题
import tensorflow.compat.v1 as tf tf.disable_v2_behavior()
这行代码的作用是导入TensorFlow的v1版本,并禁用v2版本的行为。
TensorFlow是一个流行的深度学习框架,目前已经发展到了v2版本。然而,v2版本有一些不兼容v1版本的变化,因此有时需要使用v1版本来运行旧的代码。
在这里,使用"as"关键字给TensorFlow的v1版本起了一个别名"tf"。接下来,调用"disable_v2_behavior()"函数来禁用TensorFlow的v2行为,这意味着在这个环境中,只能使用v1版本的TensorFlow代码。
import tensorflow.compat.v1 as tf ModuleNotFoundError: No module named 'tensorflow.compat'
这个错误通常是因为你的TensorFlow版本不兼容所导致的。你可以尝试以下两种解决方法:
1.卸载当前的TensorFlow版本并安装与你的代码兼容的版本。你可以使用以下命令卸载TensorFlow:
```
pip uninstall tensorflow
```
然后使用以下命令安装与你的代码兼容的版本:
```
pip install tensorflow==2.3.1 -i https://pypi.douban.com/simple
```
2.按照博客所述,将`import tensorflow as tf`改为`import tensorflow.compat.v1 as tf`。这将使你的代码与TensorFlow 1.x版本兼容。
```python
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
```
阅读全文
相关推荐
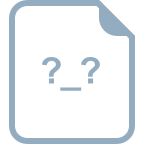
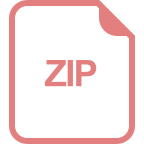
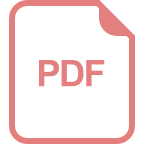











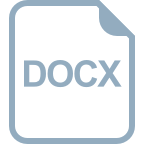
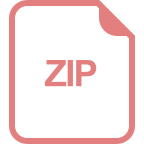
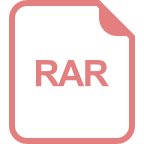