vue3中如何调用一个封装的函数
时间: 2023-05-17 09:06:25 浏览: 161
在 Vue3 中,可以通过在组件中使用 `setup()` 函数来调用封装的函数。首先,需要将封装的函数导出,然后在组件中引入该函数,并在 `setup()` 函数中调用它。例如:
```javascript
// 封装的函数
export function myFunction() {
// do something
}
// 组件中引入函数
import { myFunction } from './utils';
export default {
setup() {
// 调用函数
myFunction();
}
}
```
注意,`setup()` 函数只能在 Vue3 中使用,如果你正在使用 Vue2 或更早的版本,则需要使用不同的方法来调用封装的函数。
相关问题
vue3项目控制按钮 封装函数的方法
你可以使用Vue.js提供的自定义指令来封装控制按钮的函数。以下是一个示例:
```javascript
// 在Vue实例中定义一个自定义指令
Vue.directive('button-control', {
bind: function(el, binding) {
// 在绑定时获取指令的参数
const { value } = binding;
// 在按钮上绑定点击事件
el.addEventListener('click', () => {
// 执行传入的函数
value();
});
// 根据传入的参数控制按钮的可用状态
el.disabled = binding.arg === 'disable';
}
});
```
在模板中使用这个自定义指令:
```html
<template>
<button v-button-control:disable="disableBtn" v-button-control="handleClick">点击我</button>
</template>
```
这里的`v-button-control:disable`指令参数中,`:disable`表示将`disableBtn`的值作为参数传入指令,指令可以根据参数值来控制按钮的可用状态。`v-button-control`指令参数中,`handleClick`表示要执行的函数。
如何用vue3封装调用函数式的组件
在Vue 3中,可以使用`defineComponent`函数来封装调用函数式的组件。下面是一个简单的示例:
```javascript
import { defineComponent } from 'vue';
// 定义一个函数式组件
const FunctionalComponent = (props, context) => {
// 函数式组件逻辑
return () => {
// 返回渲染的内容
// 注意:需要使用`.value`来访问props和context
return <div>{props.msg}</div>;
};
};
// 封装调用函数式组件
const FunctionalWrapper = defineComponent({
setup() {
// 在setup函数中返回函数式组件
return FunctionalComponent;
},
});
export default FunctionalWrapper;
```
在上面的示例中,我们首先定义了一个函数式组件`FunctionalComponent`,它接受`props`和`context`作为参数,并返回一个渲染函数。然后,我们使用`defineComponent`来封装调用这个函数式组件,并将其作为`setup`函数的返回值。
在封装的组件中,我们可以像普通组件一样使用它,例如:
```html
<template>
<FunctionalWrapper msg="Hello Vue 3!" />
</template>
```
这样就可以封装和调用函数式组件了。注意,在使用函数式组件时,需要使用`.value`来访问`props`和`context`,因为Vue 3对响应性做了一些改变。
阅读全文
相关推荐
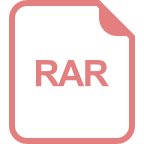







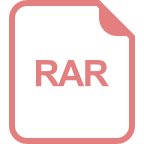




