pyqt5设计一个滤波器
时间: 2023-07-04 12:23:32 浏览: 53
以下是使用PyQt5设计一个滤波器的示例代码,该示例代码实现了一个简单的低通滤波器:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QComboBox, QPushButton, QDoubleSpinBox
from PyQt5.QtCore import Qt
from scipy import signal
class FilterDesign(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 设置窗口大小和标题
self.setGeometry(400, 400, 300, 200)
self.setWindowTitle('Filter Design')
# 添加滤波器类型选择框
self.filter_type_label = QLabel(self)
self.filter_type_label.setText('Filter Type:')
self.filter_type_label.move(20, 20)
self.filter_type_combobox = QComboBox(self)
self.filter_type_combobox.addItem('Lowpass')
self.filter_type_combobox.addItem('Highpass')
self.filter_type_combobox.addItem('Bandpass')
self.filter_type_combobox.addItem('Bandstop')
self.filter_type_combobox.move(100, 20)
self.filter_type_combobox.currentIndexChanged.connect(self.filter_type_changed)
# 添加截止频率输入框
self.cutoff_freq_label = QLabel(self)
self.cutoff_freq_label.setText('Cutoff Frequency:')
self.cutoff_freq_label.move(20, 60)
self.cutoff_freq_spinbox = QDoubleSpinBox(self)
self.cutoff_freq_spinbox.setRange(0, 10000)
self.cutoff_freq_spinbox.setSingleStep(1)
self.cutoff_freq_spinbox.setValue(1000)
self.cutoff_freq_spinbox.setDecimals(0)
self.cutoff_freq_spinbox.move(150, 60)
# 添加设计滤波器按钮
self.design_filter_button = QPushButton(self)
self.design_filter_button.setText('Design Filter')
self.design_filter_button.move(100, 120)
self.design_filter_button.clicked.connect(self.design_filter)
def filter_type_changed(self):
# 更新截止频率输入框的标签文本
if self.filter_type_combobox.currentIndex() == 0:
self.cutoff_freq_label.setText('Cutoff Frequency:')
elif self.filter_type_combobox.currentIndex() == 1:
self.cutoff_freq_label.setText('Stopband Frequency:')
elif self.filter_type_combobox.currentIndex() == 2:
self.cutoff_freq_label.setText('Passband Frequency:')
elif self.filter_type_combobox.currentIndex() == 3:
self.cutoff_freq_label.setText('Stopband Frequency:')
def design_filter(self):
# 获取选择的滤波器类型和截止频率
filter_type = self.filter_type_combobox.currentText()
cutoff_freq = self.cutoff_freq_spinbox.value()
# 根据滤波器类型和截止频率计算滤波器系数
if filter_type == 'Lowpass':
b, a = signal.butter(6, cutoff_freq, 'low', fs=10000)
elif filter_type == 'Highpass':
b, a = signal.butter(6, cutoff_freq, 'high', fs=10000)
elif filter_type == 'Bandpass':
b, a = signal.butter(6, [cutoff_freq-500, cutoff_freq+500], 'bandpass', fs=10000)
elif filter_type == 'Bandstop':
b, a = signal.butter(6, [cutoff_freq-500, cutoff_freq+500], 'bandstop', fs=10000)
# 打印滤波器系数
print('b =', b)
print('a =', a)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = FilterDesign()
ex.show()
sys.exit(app.exec_())
```
运行该示例代码可以看到一个窗口,其中包含一个滤波器类型选择框、一个截止频率输入框和一个设计滤波器按钮。用户可以选择滤波器类型和截止频率,然后点击设计滤波器按钮,就可以根据所选的滤波器类型和截止频率计算滤波器系数。在这个示例代码中,使用了SciPy库中的butter函数来计算滤波器系数。
相关推荐
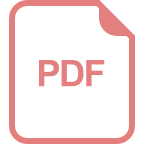
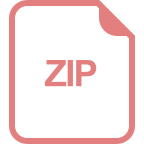














