C sharp 快速排序完整代码
时间: 2023-09-24 14:06:19 浏览: 180
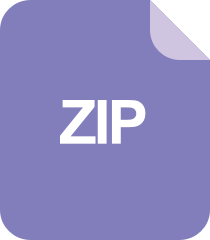
快速排序的完整代码
### 回答1:
C#中快速排序的完整代码如下:
```
using System;
using System.Linq;
class QuickSort
{
static void Main(string[] args)
{
int[] arr = { 10, 7, 8, 9, 1, 5 };
int n = arr.Length;
QuickSort_Recursive(arr, 0, n - 1);
Console.WriteLine("Sorted array:");
Console.WriteLine(string.Join(" ", arr));
}
static void QuickSort_Recursive(int[] arr, int start, int end)
{
if (start < end)
{
int partitionIndex = Partition(arr, start, end);
QuickSort_Recursive(arr, start, partitionIndex - 1);
QuickSort_Recursive(arr, partitionIndex + 1, end);
}
}
static int Partition(int[] arr, int start, int end)
{
int pivot = arr[end];
int i = (start - 1);
for (int j = start; j < end; j++)
{
if (arr[j] <= pivot)
{
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp1 = arr[i + 1];
arr[i + 1] = arr[end];
arr[end] = temp1;
return i + 1;
}
}
```
请注意,这段代码是一个控制台应用程序,需要使用Visual Studio或其他类似工具编译运行。
### 回答2:
下面是C#中的一个快速排序的完整代码实现:
```csharp
using System;
class QuickSort {
static void Main(string[] args) {
int[] array = { 5, 12, 2, 18, 9, 7, 15, 3 };
Console.WriteLine("排序前的数组:");
PrintArray(array);
QuickSortAlgorithm(array, 0, array.Length - 1);
Console.WriteLine("排序后的数组:");
PrintArray(array);
}
static void QuickSortAlgorithm(int[] array, int left, int right) {
if (left < right) {
int pivotIndex = Partition(array, left, right);
QuickSortAlgorithm(array, left, pivotIndex - 1);
QuickSortAlgorithm(array, pivotIndex + 1, right);
}
}
static int Partition(int[] array, int left, int right) {
int pivot = array[right];
int i = left - 1;
for (int j = left; j < right; j++) {
if (array[j] <= pivot) {
i++;
Swap(array, i, j);
}
}
Swap(array, i + 1, right);
return i + 1;
}
static void Swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
static void PrintArray(int[] array) {
for (int i = 0; i < array.Length; i++) {
Console.Write("{0} ", array[i]);
}
Console.WriteLine();
}
}
```
这段代码首先定义一个`QuickSort`类,并在`Main`方法中初始化一个整型数组。然后调用`QuickSortAlgorithm`方法实现快速排序,并在排序前后分别输出数组内容。每个方法的功能如下:
- `QuickSortAlgorithm`方法递归地利用`Partition`方法进行排序。如果左边索引小于右边索引,则选择一个枢纽(pivot)元素并调用`Partition`方法将数组划分为两个子数组,然后再对子数组分别递归调用`QuickSortAlgorithm`方法进行排序。
- `Partition`方法选择一个枢纽元素,将小于枢纽元素的元素放在枢纽元素左边,大于枢纽元素的元素放在右边,最后返回枢纽元素的索引。
- `Swap`方法用于交换数组中的两个元素。
- `PrintArray`方法用于输出数组的内容。
最后,程序运行时会按顺序先输出排序前的数组,然后输出排序后的数组。
### 回答3:
C#快速排序是一种常用的排序算法,它通过选择一个基准元素,将数组分成两个子数组,然后对子数组进行递归排序。以下是C#实现快速排序的完整代码:
```csharp
using System;
public class QuickSort
{
public static void Main(string[] args)
{
int[] array = { 5, 9, 3, 1, 8, 6, 2, 7, 4 };
Console.WriteLine("排序前的数组:");
PrintArray(array);
QuickSortAlgorithm(array, 0, array.Length - 1);
Console.WriteLine("排序后的数组:");
PrintArray(array);
}
static void QuickSortAlgorithm(int[] array, int left, int right)
{
if(left < right)
{
int pivot = Partition(array, left, right);
if(pivot > 1)
{
QuickSortAlgorithm(array, left, pivot - 1);
}
if(pivot + 1 < right)
{
QuickSortAlgorithm(array, pivot + 1, right);
}
}
}
static int Partition(int[] array, int left, int right)
{
int pivot = array[left];
while(true)
{
while(array[left] < pivot)
{
left++;
}
while(array[right] > pivot)
{
right--;
}
if(left < right)
{
if(array[left] == array[right])
{
return right;
}
int temp = array[left];
array[left] = array[right];
array[right] = temp;
}
else
{
return right;
}
}
}
static void PrintArray(int[] array)
{
foreach(int num in array)
{
Console.Write(num + " ");
}
Console.WriteLine();
}
}
```
这段代码中,我们首先定义了一个数组,然后调用`QuickSortAlgorithm`函数进行排序,并输出排序前后的数组。`QuickSortAlgorithm`函数使用递归方式实现快速排序,它通过选择基准元素并将数组分成两个子数组,分别对子数组进行排序。`Partition`函数用于确定基准元素的位置,并将小于基准元素的值放在左边,大于基准元素的值放在右边。最后,我们通过`PrintArray`函数打印出排序后的数组。
阅读全文
相关推荐

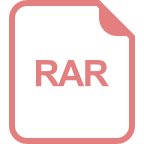

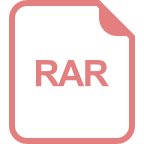
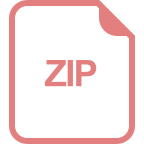
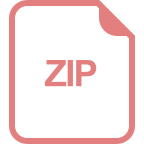
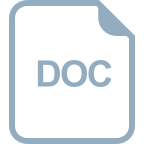
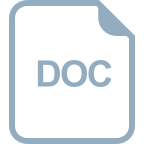
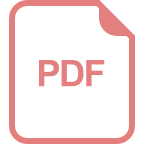
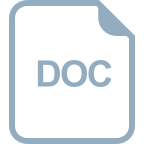
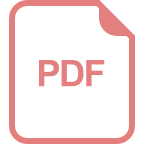
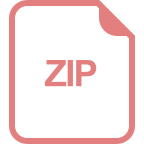
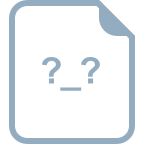
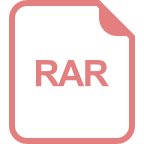
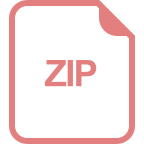