c# 调用带参数的exe
时间: 2023-07-31 19:05:45 浏览: 68
在 C# 中调用带参数的可执行文件,可以使用 `Process.Start` 方法。下面是一个简单的例子:
假设我们有一个名为 `hello.exe` 的可执行文件,它接收一个参数:
```csharp
using System;
using System.Diagnostics;
class Program
{
static void Main(string[] args)
{
Process.Start("hello.exe", "Tom");
}
}
```
在这个例子中,我们使用 `Process.Start` 方法调用 `hello.exe`,并传入参数 "Tom"。
如果需要传入多个参数,可以使用空格分隔它们,例如:
```csharp
Process.Start("hello.exe", "Tom 18");
```
此时,`hello.exe` 将接收两个参数,分别为 "Tom" 和 "18"。
如果需要在 C# 中获取可执行文件的输出结果,可以使用 `Process.StandardOutput` 属性。例如:
```csharp
using System;
using System.Diagnostics;
class Program
{
static void Main(string[] args)
{
Process process = new Process();
process.StartInfo.FileName = "hello.exe";
process.StartInfo.Arguments = "Tom";
process.StartInfo.RedirectStandardOutput = true;
process.StartInfo.UseShellExecute = false;
process.Start();
string output = process.StandardOutput.ReadToEnd();
process.WaitForExit();
Console.WriteLine(output);
}
}
```
在这个例子中,我们创建了一个 `Process` 对象,设置了 `FileName` 和 `Arguments` 属性,以及 `RedirectStandardOutput` 和 `UseShellExecute` 属性。然后调用 `Start` 方法启动进程,并使用 `StandardOutput` 属性获取输出结果。最后调用 `WaitForExit` 方法等待进程执行完毕,并输出输出结果。
相关推荐
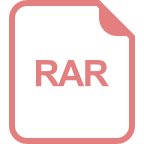














