写一个springboot+vue文件下载
时间: 2023-12-26 15:50:25 浏览: 85
以下是一个基于Spring Boot和Vue.js的文件下载示例:
1. 创建Spring Boot项目
首先,我们需要创建一个Spring Boot项目。可以使用Spring Initializr快速创建一个空的Spring Boot项目。我们需要添加以下依赖项:
- web:用于构建Web应用程序
- jpa:用于与数据库交互
2. 创建文件下载服务
接下来,我们需要创建一个文件下载服务。我们可以使用Spring Boot的控制器来实现这一点。以下是一个简单的示例:
```java
@RestController
public class FileDownloadController {
private final ResourceLoader resourceLoader;
public FileDownloadController(ResourceLoader resourceLoader) {
this.resourceLoader = resourceLoader;
}
@GetMapping("/download")
public ResponseEntity<Resource> downloadFile(@RequestParam("file") String fileName) {
Resource resource = resourceLoader.getResource("classpath:static/" + fileName);
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
}
}
```
在这个示例中,我们通过@RequestParam注释接收文件名参数。然后,我们使用ResourceLoader从classpath:static目录加载文件资源。最后,我们将文件资源包装在ResponseEntity中,并设置文件名和Content-Disposition标头。
3. 创建Vue.js前端
接下来,我们需要创建Vue.js前端。我们将使用axios库从服务器下载文件。以下是一个简单的示例:
```html
<template>
<div>
<h1>File Download</h1>
<form @submit.prevent="downloadFile">
<label for="fileName">File Name:</label>
<input type="text" id="fileName" v-model="fileName">
<button type="submit">Download</button>
</form>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
fileName: ''
};
},
methods: {
downloadFile() {
const url = '/download?file=' + this.fileName;
axios.get(url, { responseType: 'blob' })
.then(response => {
const url = window.URL.createObjectURL(new Blob([response.data]));
const link = document.createElement('a');
link.href = url;
link.setAttribute('download', this.fileName);
document.body.appendChild(link);
link.click();
})
.catch(error => {
console.error(error);
});
}
}
};
</script>
```
在这个示例中,我们创建了一个表单,用于输入文件名。然后,我们使用axios库从服务器下载文件。我们将响应类型设置为“blob”,以便我们可以将文件保存到本地。最后,我们创建一个链接,并模拟点击它,以便下载文件。
4. 运行应用程序
现在,我们可以将应用程序运行起来。使用以下命令:
```
./mvnw spring-boot:run
```
应用程序将在端口8080上启动。
5. 测试应用程序
现在,我们可以测试应用程序。打开浏览器,输入以下URL:
```
http://localhost:8080/
```
应用程序将显示一个文件下载表单。输入文件名并点击下载按钮。文件将自动下载到本地计算机。
阅读全文
相关推荐
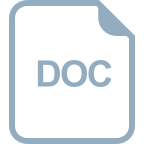
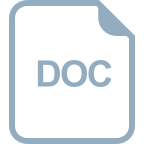
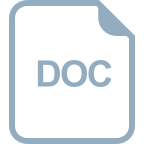
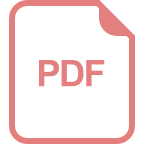
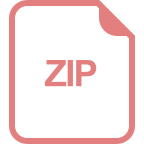
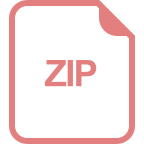
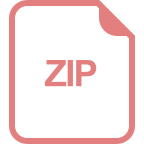
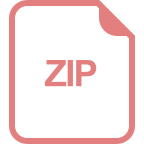
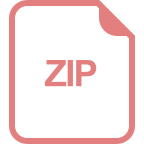
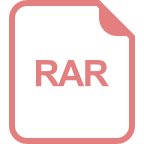
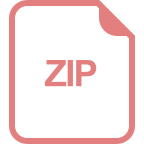
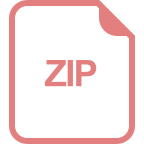
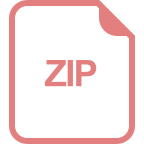
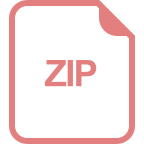
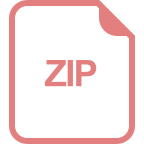
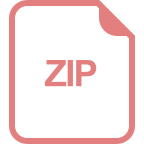
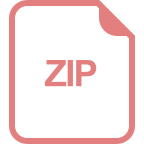
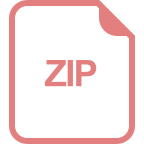