java实现文件分片上传并且断点续传
时间: 2023-08-16 16:09:04 浏览: 77
实现文件分片上传并且断点续传的一种常见方案是使用HTTP协议,将大文件进行分片上传,每个分片的大小可以根据具体情况设置,通常是几十KB到几百KB不等。上传过程中,服务器接收到每个分片后,将其存储到磁盘上。
同时,为了实现断点续传,客户端需要在上传前检查服务器上是否已经存在相同的文件。如果已存在,则客户端需要向服务器发送一个请求,以获取已上传分片的信息,然后继续上传未上传的分片。
下面是使用Java语言实现文件分片上传并且断点续传的示例代码:
```java
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
public class FileUploader {
private static final String BOUNDARY = "----WebKitFormBoundary7MA4YWxkTrZu0gW";
public static void main(String[] args) throws Exception {
String filePath = "C:\\test\\largeFile.zip";
String url = "http://localhost:8080/upload";
File file = new File(filePath);
long fileSize = file.length();
int chunkSize = 1024 * 1024; // 1MB
int chunkCount = (int) Math.ceil((double) fileSize / chunkSize);
for (int i = 0; i < chunkCount; i++) {
int start = i * chunkSize;
int end = (i + 1) * chunkSize;
if (end > fileSize) {
end = (int) fileSize;
}
uploadChunk(url, file.getName(), i, chunkCount, start, end, file);
}
}
private static void uploadChunk(String url, String fileName, int chunkIndex, int chunkCount, int start, int end, File file) throws Exception {
URL uploadUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) uploadUrl.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=" + BOUNDARY);
DataOutputStream outputStream = new DataOutputStream(connection.getOutputStream());
outputStream.writeBytes("--" + BOUNDARY + "\r\n");
outputStream.writeBytes("Content-Disposition: form-data; name=\"file\"; filename=\"" + fileName + "\"\r\n");
outputStream.writeBytes("Content-Type: application/octet-stream\r\n\r\n");
FileInputStream inputStream = new FileInputStream(file);
inputStream.skip(start);
byte[] buffer = new byte[1024];
int len;
int uploadedBytes = start;
while (uploadedBytes < end && (len = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, len);
uploadedBytes += len;
}
inputStream.close();
outputStream.writeBytes("\r\n--" + BOUNDARY + "\r\n");
outputStream.writeBytes("Content-Disposition: form-data; name=\"chunkIndex\"\r\n\r\n");
outputStream.writeBytes(String.valueOf(chunkIndex) + "\r\n");
outputStream.writeBytes("--" + BOUNDARY + "\r\n");
outputStream.writeBytes("Content-Disposition: form-data; name=\"chunkCount\"\r\n\r\n");
outputStream.writeBytes(String.valueOf(chunkCount) + "\r\n");
outputStream.writeBytes("--" + BOUNDARY + "--\r\n");
outputStream.flush();
outputStream.close();
int responseCode = connection.getResponseCode();
if (responseCode != HttpURLConnection.HTTP_OK) {
throw new RuntimeException("Failed to upload chunk: " + chunkIndex);
}
}
}
```
上述代码将文件分成若干个分片,每个分片大小为1MB,然后逐个上传到服务器。其中,`uploadChunk()`方法用于上传单个分片,它将分片数据和分片信息一起发送到服务器。服务器需要根据分片信息将所有分片组合成完整的文件。
此外,为了实现断点续传,还需要在服务器端实现一个接口,用于获取已上传分片的信息,并返回给客户端。在上传前,客户端需要向服务器发送一个请求,以获取已上传分片的信息,然后继续上传未上传的分片。在上传过程中,客户端需要记录已上传的分片信息,以便在上传失败后能够恢复上传进度。
相关推荐
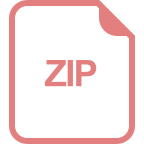
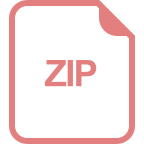













