turtle显示时间不用数码管
时间: 2024-02-20 17:57:00 浏览: 20
你可以使用Python的turtle库中的write()函数来在画布上显示时间。首先,你需要导入time模块来获取当前时间。然后,你可以使用write()函数将时间显示在画布上。下面是一个示例代码:
```
import turtle
import time
# 创建画布和画笔
canvas = turtle.Screen()
pen = turtle.Turtle()
# 设置画笔属性
pen.hideturtle()
pen.penup()
pen.goto(-50, 0)
# 循环显示时间
while True:
# 获取当前时间
current_time = time.strftime("%H:%M:%S")
# 在画布上显示时间
pen.write(current_time, font=("Arial", 24, "normal"))
# 暂停1秒钟
time.sleep(1)
# 清除上一次显示的时间
pen.clear()
```
这个代码会在画布上不断显示当前时间,并且每秒钟更新一次。你可以根据需要修改画笔的位置、字体等属性来适应你的需求。
相关问题
turtle显示日期和时间不使用数码管
你可以使用Python的turtle库和time模块来在画布上显示日期和时间。下面是一个示例代码:
```
import turtle
import time
# 创建画布和画笔
canvas = turtle.Screen()
pen = turtle.Turtle()
# 设置画笔属性
pen.hideturtle()
pen.penup()
pen.goto(-150, 0)
# 循环显示日期和时间
while True:
# 获取当前日期和时间
current_time = time.strftime("%Y-%m-%d %H:%M:%S")
# 在画布上显示日期和时间
pen.write(current_time, font=("Arial", 24, "normal"))
# 暂停1秒钟
time.sleep(1)
# 清除上一次显示的日期和时间
pen.clear()
```
这个代码会在画布上不断显示当前日期和时间,并且每秒钟更新一次。你可以根据需要修改画笔的位置、字体等属性来适应你的需求。
用python的turtle画数码管显示当前时间
好的,以下是一个用 Python 的 turtle 模块实现的数码管程序,可以显示当前时间:
```python
import turtle
import time
# 数码管字符表示
DIGITS = [
["###", "# #", "# #", "# #", "###"], # 0
[" #", " #", " #", " #", " #"], # 1
["###", " #", "###", "# ", "###"], # 2
["###", " #", "###", " #", "###"], # 3
["# #", "# #", "###", " #", " #"], # 4
["###", "# ", "###", " #", "###"], # 5
["###", "# ", "###", "# #", "###"], # 6
["###", " #", " #", " #", " #"], # 7
["###", "# #", "###", "# #", "###"], # 8
["###", "# #", "###", " #", "###"], # 9
]
# 数码管的尺寸
SIZE = 10
# 数码管的间隔
SPACE = 5
# 数码管的起始位置
START_POS = (-200, 0)
def draw_digit(digit, pos):
# 绘制一个数字的数码管
for i in range(5):
for j in range(3):
if DIGITS[digit][i][j] == "#":
x, y = pos[0] + j * SIZE, pos[1] - i * SIZE
turtle.goto(x, y)
turtle.stamp()
def show_time():
# 获取当前时间
current_time = time.strftime("%H:%M:%S")
# 将时间按每个数字拆分
digits = [int(d) for d in current_time.replace(":", "")]
# 计算数码管的总宽度
width = len(digits) * (3 * SIZE + 2 * SPACE) - SPACE
# 计算数码管的起始位置
pos = (START_POS[0] + (400 - width) / 2, START_POS[1])
# 绘制每个数字的数码管
for digit in digits:
draw_digit(digit, pos)
pos = (pos[0] + 3 * SIZE + SPACE, pos[1])
# 初始化 turtle
turtle.setup(800, 400)
turtle.penup()
turtle.shape("square")
turtle.shapesize(SIZE / 20)
turtle.color("green")
turtle.speed(0)
# 每秒更新一次时间
while True:
turtle.clearstamps()
show_time()
turtle.update()
time.sleep(1)
```
该程序使用了 turtle 模块来绘制数码管,首先定义了一个 `draw_digit` 函数来绘制一个数字的数码管,然后将当前时间转换成每个数字的拆分,计算数码管的总宽度和起始位置,最后绘制每个数字的数码管即可。为了让显示更加连续,程序使用了 turtle 的 `clearstamps` 方法来清除之前的显示。
相关推荐
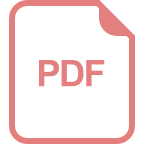







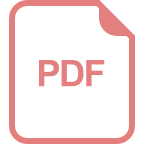
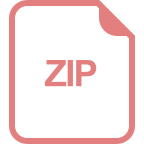
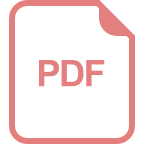
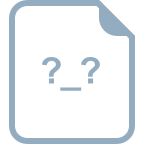