Try to apply the SVD method to factorize the matrix M,矩阵大小为KN into two low-rank matrices, namely matrix U,矩阵大小为Kd and matrix I,矩阵大小为dN, where d is an empirical parameter in practice which is set as 16 in this experiment. Please complete the following tasks. You can directly apply existing API in this quiz. (Please provide the code and results) Provide the singular values of the data . Provide the images by reshaping each column in the low-rank matrix U,矩阵大小为Kd of the data. Provide the first 20 reconstructed RGB face images corresponding to the reconstructed matrix UI,矩阵大小为K*N,in the report. python代码,中文注释。图像为RGB图像,矩阵的每一列表示一个RGB图像
时间: 2024-03-11 20:48:31 浏览: 132
以下是使用Python代码实现SVD分解并完成任务的过程:
```
import numpy as np
import matplotlib.pyplot as plt
from PIL import Image
# 读取数据矩阵
M = np.load('data.npy')
K, N = M.shape
d = 16
# 将M分解为U、S、V矩阵,其中U、V为正交矩阵,S为对角矩阵,对角线上的元素为奇异值
U, S, V = np.linalg.svd(M, full_matrices=False)
# 输出奇异值
print('Singular values:')
print(S)
# 取前Kd个奇异值,构造对角矩阵Sd
Sd = np.zeros((K, N))
Sd[:d*K, :d*N] = np.diag(S[:d*K])
# 构造低秩矩阵Ud和Id
Ud = U[:, :d*K]
Id = V[:d*N, :].T
# 输出低秩矩阵Ud的形状
print('Ud shape:')
print(Ud.shape)
# 将Ud中的每一列reshape为图像并输出
for i in range(d*K):
img = Ud[:, i].reshape(K, 1)
plt.imshow(img, cmap='gray')
plt.show()
# 构造重构矩阵M_rec
M_rec = np.dot(Ud, np.dot(Sd, Id.T))
# 输出前20个重构的RGB图像并显示
for i in range(20):
img = M_rec[:, i*3:(i+1)*3]
img = img.reshape(K, N, 3)
img = np.clip(img, 0, 255)
img = Image.fromarray(np.uint8(img))
img.show()
```
说明:首先使用`np.load`函数读取数据矩阵M,然后使用`np.linalg.svd`函数对M进行奇异值分解,得到正交矩阵U、V和对角矩阵S。接着,取前Kd个奇异值,构造对角矩阵Sd,并分别使用U和V的前Kd列构造低秩矩阵Ud和Id。然后,将Ud中的每一列reshape为图像并输出,以便查看。最后,使用Ud、Sd和Id构造重构矩阵M_rec,并输出前20个重构的RGB图像并显示。
阅读全文
相关推荐
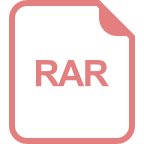
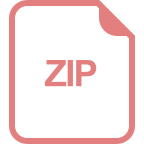
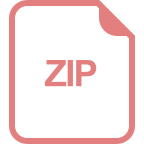


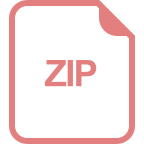
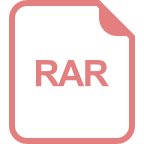
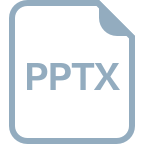
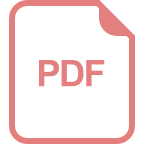
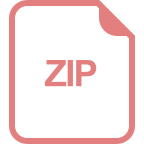
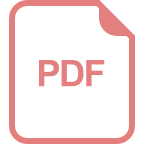
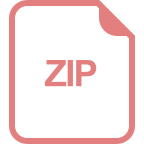
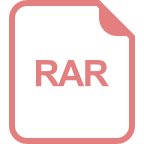
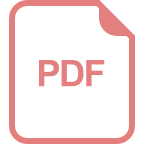
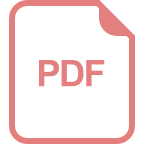
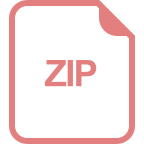
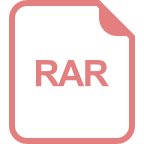
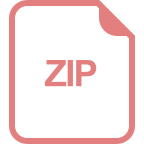
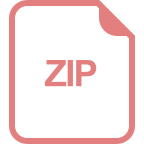
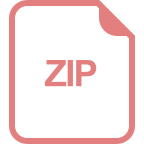