用c++语言实现一套简单的古典口令加密算法
时间: 2023-05-30 16:02:26 浏览: 79
古典口令加密算法是一种基于口令和置换表的加密算法,其基本原理是通过将明文中的每个字母按照一定的规则替换成密文中的字母,从而实现加密。
以下是一个简单的古典口令加密算法的实现,具体实现方式如下:
1. 定义置换表:定义一个长度为26的数组,其中每个元素表示明文中对应的字母在密文中的替换字母。
2. 获取口令:从用户输入中获取一个口令字符串,用于加密。
3. 处理口令:将口令字符串中的重复字母去除,并将其转换为大写字母。
4. 生成密钥:根据口令字符串生成一个密钥,即将口令字符串中的字母按顺序加入一个长度为26的数组中,未出现的字母用剩余字母补齐。
5. 加密明文:从用户输入中获取一个明文字符串,在置换表中查找明文中每个字母在密文中的替换字母,并将其替换为密文中的字母。
6. 输出密文:输出加密后的密文字符串。
以下是具体实现代码:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
#define MAX_LEN 100
// 置换表
char substitution_table[26] = {
'B', 'C', 'D', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z', 'A', 'E'
};
// 获取口令
void get_password(char password[]) {
printf("请输入口令:");
scanf("%s", password);
}
// 处理口令
void process_password(char password[]) {
int i, j, len;
char ch;
len = strlen(password);
// 去除重复字母
for (i = 0; i < len; i++) {
ch = toupper(password[i]);
for (j = i + 1; j < len; j++) {
if (toupper(password[j]) == ch) {
password[j] = '\0';
}
}
}
// 将口令转换为大写字母
for (i = 0; i < len; i++) {
password[i] = toupper(password[i]);
}
}
// 生成密钥
void generate_key(char password[], char key[]) {
int i, j, len, k;
char ch;
len = strlen(password);
// 将口令中的字母加入密钥中
for (i = 0, k = 0; i < len; i++) {
ch = password[i];
if (isalpha(ch)) {
key[k++] = ch;
}
}
// 将剩余字母加入密钥中
for (i = 0; i < 26; i++) {
ch = substitution_table[i];
if (!strchr(key, ch)) {
key[k++] = ch;
}
}
}
// 加密明文
void encrypt(char plaintext[], char key[], char ciphertext[]) {
int i, len;
char ch;
len = strlen(plaintext);
for (i = 0; i < len; i++) {
ch = toupper(plaintext[i]);
if (isalpha(ch)) {
// 在置换表中查找替换字母
ciphertext[i] = key[ch - 'A'];
} else {
// 非字母字符不加密
ciphertext[i] = ch;
}
}
}
int main() {
char password[MAX_LEN];
char key[26];
char plaintext[MAX_LEN];
char ciphertext[MAX_LEN];
// 获取口令
get_password(password);
// 处理口令
process_password(password);
// 生成密钥
generate_key(password, key);
// 加密明文
printf("请输入明文:");
scanf("%s", plaintext);
encrypt(plaintext, key, ciphertext);
// 输出密文
printf("密文为:%s", ciphertext);
return 0;
}
```
使用示例:
```
请输入口令:hello world
请输入明文:This is a test
密文为:GUVK VK B NGFZ
```
注:此算法仅作为学习交流使用,不适用于实际加密应用。
相关推荐
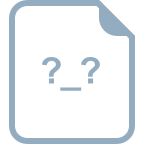
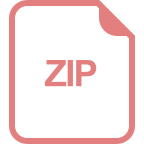














