编写程序使用Mediapipe 和opencv实现手指数量的识别
时间: 2024-02-21 18:59:52 浏览: 25
下面是使用Mediapipe和OpenCV实现手指数量识别的Python代码:
```python
import cv2
import mediapipe as mp
# 初始化mediapipe手部模型
mp_hands = mp.solutions.hands
hands = mp_hands.Hands()
# 初始化计数器和状态
count = 0
tipIds = [4, 8, 12, 16, 20]
hand_status = ""
# 打开摄像头
cap = cv2.VideoCapture(0)
while cap.isOpened():
success, frame = cap.read()
if not success:
print("Ignoring empty camera frame.")
continue
# 将图像从BGR转换为RGB
image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 检测手
results = hands.process(image)
# 获取手部landmarks
landmarks = []
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
for lm in hand_landmarks.landmark:
landmarks.append(lm)
# 计算手指数量
fingers = []
if len(landmarks) > 0:
for id in tipIds:
x = landmarks[id].x
y = landmarks[id].y
if landmarks[id - 2].x > x:
fingers.append(1)
else:
fingers.append(0)
count = fingers.count(1)
# 更新手部状态
if count == 0:
hand_status = "Closed"
elif count == 5:
hand_status = "Open"
else:
hand_status = "Unknown"
# 将手指数量和状态显示在图像上
cv2.putText(frame, f'Fingers: {count}', (10, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2, cv2.LINE_AA)
cv2.putText(frame, f'Status: {hand_status}', (10, 100), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2, cv2.LINE_AA)
# 显示图像
cv2.imshow("Hand Gesture Recognition", frame)
# 按下q键退出程序
if cv2.waitKey(5) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
这段代码使用了Mediapipe和OpenCV库,通过摄像头获取图像,并调用Mediapipe的手部模型检测手部landmarks,然后根据手指的终点和起点的位置来计算手指数量,最后将手指数量和手部状态显示在图像上。用户可以在程序运行过程中通过摄像头展示手势,程序会实时计算出手指的数量和手部状态,并在图像上进行显示。
相关推荐
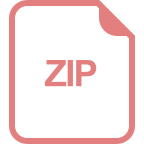
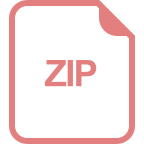
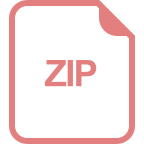









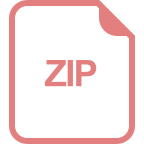
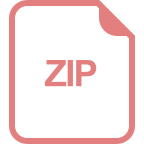
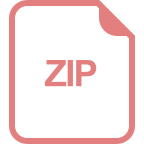
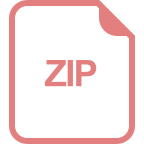
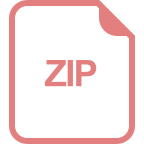