在WPF中,用Livecharts实现动态曲线
时间: 2024-02-11 08:09:13 浏览: 254
使用LiveCharts实现动态曲线的步骤如下:
1. 在Visual Studio中创建一个WPF项目。
2. 在NuGet包管理器中搜索并安装LiveCharts.Wpf包。
3. 在MainWindow.xaml中添加一个LineChart控件。
```xml
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:lvc="clr-namespace:LiveCharts.Wpf;assembly=LiveCharts.Wpf"
Title="MainWindow" Height="350" Width="525">
<Grid>
<lvc:CartesianChart Name="Chart">
<lvc:CartesianChart.Series>
<lvc:LineSeries Name="Series" Values="{Binding LineValues}" />
</lvc:CartesianChart.Series>
</lvc:CartesianChart>
</Grid>
</Window>
```
4. 在MainWindow.xaml.cs中定义一个ViewModel类,用于绑定数据到LineChart控件上。
```csharp
public class ViewModel : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
private ChartValues<double> _lineValues;
public ChartValues<double> LineValues
{
get { return _lineValues; }
set
{
_lineValues = value;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs("LineValues"));
}
}
public ViewModel()
{
LineValues = new ChartValues<double>();
Timer timer = new Timer(1000);
timer.Elapsed += Timer_Elapsed;
timer.Start();
}
private void Timer_Elapsed(object sender, ElapsedEventArgs e)
{
Random random = new Random();
double value = random.Next(0, 100);
LineValues.Add(value);
}
}
```
5. 在MainWindow.xaml.cs的构造函数中创建ViewModel实例,并将其设置为DataContext。
```csharp
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
DataContext = new ViewModel();
}
}
```
6. 运行程序,即可看到动态曲线。
通过上述步骤,我们可以使用LiveCharts在WPF中实现动态曲线。其中,关键是使用ChartValues类来绑定数据到LineChart控件上,并使用Timer类来定时更新数据。
阅读全文
相关推荐
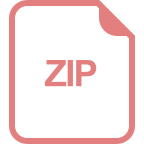
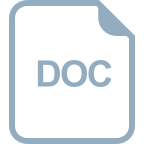

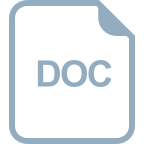
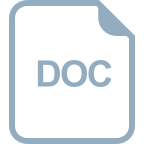
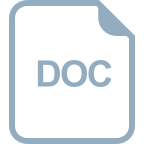






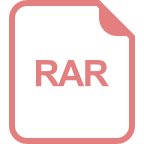
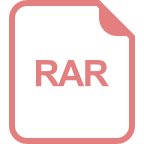
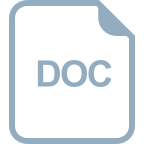