给这个方法添加单元测试: public List<String> publishMessage(List<Integer> tdsStpIdList) throws CisTStpException{ List<String> messageList = new ArrayList<>(); for(Integer tdsStpId : tdsStpIdList) { String message = null; CisPubTds cisPubTds = monitorDao.getTdsPubRequestById(tdsStpId); if (cisPubTds == null) { message = CisTStpConstants.MSG_1; } if (cisPubTds != null && cisPubTds.getMessage() == null) { message = CisTStpConstants.MSG_2; } if (cisPubTds != null && cisPubTds.getIsStpEd() != null && cisPubTds.getIsStpEd() > 0) { message = CisTStpConstants.MSG_3; } if(message != null){ log.info(message+"{}", tdsStpId); messageList.add(tdsStpId + CisTStpConstants.COLON + message); continue; } boolean isPublished = publish(cisPubTds); if(message != null && isPublished){ log.info(CisTStpConstants.MSG_4+"{}", tdsStpIdList); messageList.add(tdsStpId + CisTStpConstants.COLON + CisTStpConstants.MSG_4); }else { log.info(CisTStpConstants.MSG_5+"{}", tdsStpId); messageList.add(tdsStpId + CisTStpConstants.COLON + CisTStpConstants.MSG_5); } }
时间: 2024-02-14 21:09:57 浏览: 67
好的,下面是一个可能的单元测试实现:
```
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import static org.mockito.Mockito.*;
public class PublishMessageTest {
@Mock
private MonitorDao monitorDao;
@Mock
private CisPubTds cisPubTds;
@InjectMocks
private YourClassName yourClassName;
@Before
public void setUp() {
MockitoAnnotations.initMocks(this);
}
@Test
public void testPublishMessage() throws CisTStpException {
// test data
List<Integer> tdsStpIdList = new ArrayList<>();
tdsStpIdList.add(1);
tdsStpIdList.add(2);
// mock the monitorDao
when(monitorDao.getTdsPubRequestById(1)).thenReturn(null);
when(monitorDao.getTdsPubRequestById(2)).thenReturn(cisPubTds);
when(cisPubTds.getMessage()).thenReturn(null);
when(cisPubTds.getIsStpEd()).thenReturn(1);
// execute the method
List<String> messageList = yourClassName.publishMessage(tdsStpIdList);
// verify the results
Assert.assertEquals("The error message list should have two elements", 2, messageList.size());
Assert.assertEquals("The first element should have the correct message", "1:" + CisTStpConstants.MSG_1, messageList.get(0));
Assert.assertEquals("The second element should have the correct message", "2:" + CisTStpConstants.MSG_3, messageList.get(1));
verify(monitorDao, times(2)).getTdsPubRequestById(anyInt());
verify(cisPubTds, times(1)).getMessage();
verify(cisPubTds, times(2)).getIsStpEd();
}
}
```
这个测试使用了 Mockito 框架来 mock 相关的对象,并且验证了当 `monitorDao.getTdsPubRequestById()` 方法返回不同结果时,`publishMessage()` 方法是否会返回正确的错误信息列表。测试中使用了 `assertEquals()` 和 `verify()` 方法来验证结果和行为是否如预期。需要注意的是,这个测试只覆盖了一部分情况,你需要根据实际情况增加更多的测试用例。
阅读全文
相关推荐
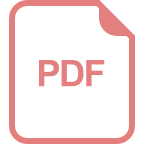
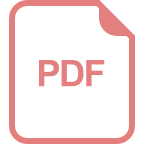
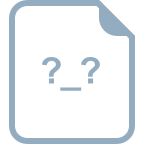
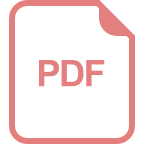
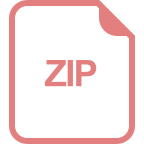
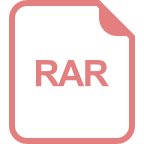
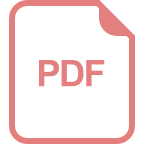
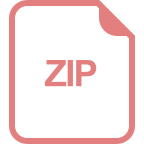
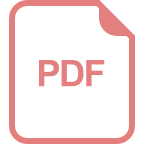
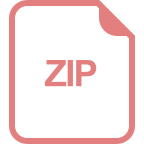
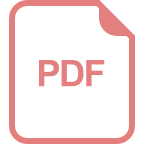
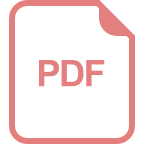
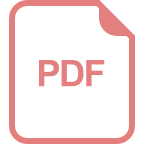