java部署在服务器上,如何从另外一台服务器中复制文件夹到本地服务器
时间: 2023-05-11 20:04:12 浏览: 117
您可以使用SCP命令将文件夹从远程服务器复制到本地服务器。SCP命令的语法如下:
scp -r username@remote:/path/to/folder /path/to/local/folder
其中,username是远程服务器的用户名,remote是远程服务器的IP地址或域名,/path/to/folder是要复制的文件夹的路径,/path/to/local/folder是本地服务器上要保存文件夹的路径。-r选项表示递归复制整个文件夹。
相关问题
Java程序部署在服务器上,如何用java让服务器从另一台服务器上复制一个文件夹
您可以使用Java中的File类和IO流来实现从另一台服务器上复制一个文件夹。具体步骤如下:
1. 创建一个File对象,指定要复制的文件夹路径。
2. 使用File类的listFiles()方法获取该文件夹下的所有文件和子文件夹。
3. 遍历所有文件和子文件夹,如果是文件夹则递归调用复制方法,如果是文件则使用IO流将文件复制到目标服务器上。
4. 复制完成后关闭IO流。
以下是示例代码:
```
import java.io.*;
public class CopyFolder {
public static void main(String[] args) {
String sourceFolder = "/path/to/source/folder";
String targetFolder = "/path/to/target/folder";
File source = new File(sourceFolder);
File target = new File(targetFolder);
copyFolder(source, target);
}
public static void copyFolder(File source, File target) {
if (source.isDirectory()) {
if (!target.exists()) {
target.mkdir();
}
String[] files = source.list();
for (String file : files) {
File srcFile = new File(source, file);
File tgtFile = new File(target, file);
copyFolder(srcFile, tgtFile);
}
} else {
try (InputStream in = new FileInputStream(source);
OutputStream out = new FileOutputStream(target)) {
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
java工程部署在服务器上,要将本服务器的文件夹备份到另外一台服务器中
您可以使用 rsync 命令将本服务器的文件夹备份到另外一台服务器中。具体命令如下:
rsync -avz /path/to/source/folder/ user@remote_host:/path/to/destination/folder/
其中,/path/to/source/folder/ 是本服务器上要备份的文件夹路径,user 是另外一台服务器的用户名,remote_host 是另外一台服务器的 IP 地址或域名,/path/to/destination/folder/ 是备份到另外一台服务器上的目标文件夹路径。-avz 参数表示以归档模式备份,保留文件属性和权限,并压缩传输数据,以节省带宽和时间。
阅读全文
相关推荐







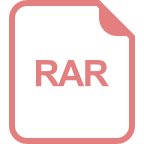








