c#(1)初始化:设定n(取值范围[5, 20])和m(取值范围[5, 20]),为n个图形随机初始化[1, n]个数字,并按数字升序排列图形并显示。例如当n=15时,显示结果如下: (2)专注力训练:点击开始按钮,用m*m个随机图片填满方阵中的每个位置。每个图片下方设置一个文本框,用户可以输入数字。例如m=4时,随机显示的图片方阵如下: 计时开始,并显示所用时间。 当用户点击结束按钮时,计时结束,显示本次用时和正确率(正确标准:用户输入数字与初始化时图片对应数字相同) (3)历史记录:查询不同n和m时,历史最好成绩。查询某一n和某一m时,所有的历史成绩。
时间: 2024-02-03 11:14:22 浏览: 19
这个任务可以分为三个部分来完成:
### 初始化
1. 定义n和m变量,使用Random类生成[1, n]范围内的随机数字,并存储在数组中。
2. 对数组进行升序排序,并显示图形。
C#代码示例:
```csharp
int n = new Random().Next(5, 21); // 生成[5, 20]范围内的随机数字
int[] numbers = new int[n];
for (int i = 0; i < n; i++)
{
numbers[i] = new Random().Next(1, n + 1); // 生成[1, n]范围内的随机数字
}
Array.Sort(numbers); // 升序排序
for (int i = 0; i < n; i++)
{
Console.Write(numbers[i] + " ");
}
```
### 专注力训练
1. 定义m变量,使用Random类生成[5, 20]范围内的随机数字。
2. 使用PictureBox控件显示m * m个随机图片,并在下方设置一个文本框。
3. 点击开始按钮启动计时器,并记录开始时间。
4. 用户输入数字并检验与图片对应数字是否相同,计算正确率。
5. 点击结束按钮停止计时器,并记录结束时间和正确率。
C#代码示例:
```csharp
int m = new Random().Next(5, 21); // 生成[5, 20]范围内的随机数字
PictureBox[,] pictureBoxes = new PictureBox[m, m];
TextBox[,] textBoxes = new TextBox[m, m];
for (int i = 0; i < m; i++)
{
for (int j = 0; j < m; j++)
{
pictureBoxes[i, j] = new PictureBox();
pictureBoxes[i, j].Image = GetRandomImage(); // 获取随机图片
pictureBoxes[i, j].SizeMode = PictureBoxSizeMode.StretchImage;
pictureBoxes[i, j].Location = new Point(i * 50, j * 50);
pictureBoxes[i, j].Size = new Size(50, 50);
this.Controls.Add(pictureBoxes[i, j]);
textBoxes[i, j] = new TextBox();
textBoxes[i, j].Location = new Point(i * 50, j * 50 + 50);
textBoxes[i, j].Size = new Size(50, 20);
this.Controls.Add(textBoxes[i, j]);
}
}
DateTime startTime = DateTime.Now;
Timer timer = new Timer();
timer.Interval = 1000;
timer.Tick += Timer_Tick;
timer.Start();
double correctCount = 0;
for (int i = 0; i < m; i++)
{
for (int j = 0; j < m; j++)
{
int number = GetImageNumber(pictureBoxes[i, j].Image); // 获取图片对应数字
int inputNumber;
if (int.TryParse(textBoxes[i, j].Text, out inputNumber))
{
if (number == inputNumber)
{
correctCount++;
}
}
}
}
double accuracy = correctCount / (m * m);
void Timer_Tick(object sender, EventArgs e)
{
TimeSpan timeSpan = DateTime.Now - startTime;
Console.WriteLine("用时:" + timeSpan.TotalSeconds + "秒");
Console.WriteLine("正确率:" + accuracy);
timer.Stop();
}
```
### 历史记录
1. 使用SQLite或其他数据库存储每次训练的结果,包括n、m、用时和正确率。
2. 查询不同n和m时,返回历史最好成绩。
3. 查询某一n和某一m时,返回所有的历史成绩。
C#代码示例:
```csharp
// 初始化数据库连接
string connectionString = "Data Source=training.db";
using (SQLiteConnection connection = new SQLiteConnection(connectionString))
{
connection.Open();
// 创建表
using (SQLiteCommand command = new SQLiteCommand("CREATE TABLE IF NOT EXISTS Training (Id INTEGER PRIMARY KEY AUTOINCREMENT, N INTEGER, M INTEGER, Time REAL, Accuracy REAL)", connection))
{
command.ExecuteNonQuery();
}
// 插入数据
using (SQLiteCommand command = new SQLiteCommand("INSERT INTO Training (N, M, Time, Accuracy) VALUES (@N, @M, @Time, @Accuracy)", connection))
{
command.Parameters.AddWithValue("@N", n);
command.Parameters.AddWithValue("@M", m);
command.Parameters.AddWithValue("@Time", timeSpan.TotalSeconds);
command.Parameters.AddWithValue("@Accuracy", accuracy);
command.ExecuteNonQuery();
}
// 查询不同n和m的历史最好成绩
using (SQLiteCommand command = new SQLiteCommand("SELECT N, M, MIN(Time) AS BestTime, MAX(Accuracy) AS BestAccuracy FROM Training GROUP BY N, M", connection))
{
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
int n = reader.GetInt32(0);
int m = reader.GetInt32(1);
double bestTime = reader.GetDouble(2);
double bestAccuracy = reader.GetDouble(3);
Console.WriteLine("n={0}, m={1}, 历史最好成绩:用时={2}秒,正确率={3}", n, m, bestTime, bestAccuracy);
}
}
}
// 查询某一n和某一m的所有历史成绩
using (SQLiteCommand command = new SQLiteCommand("SELECT Time, Accuracy FROM Training WHERE N=@N AND M=@M", connection))
{
command.Parameters.AddWithValue("@N", 10);
command.Parameters.AddWithValue("@M", 10);
using (SQLiteDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
double time = reader.GetDouble(0);
double accuracy = reader.GetDouble(1);
Console.WriteLine("用时={0}秒,正确率={1}", time, accuracy);
}
}
}
}
```
相关推荐
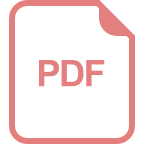
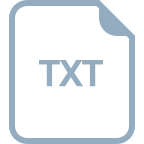
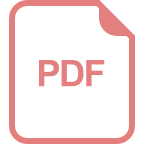














