python制表符对不齐怎么办
时间: 2023-05-28 14:05:08 浏览: 56
如果Python代码中的制表符对不齐,可以尝试使用空格进行对齐。在Python中,建议使用空格代替制表符进行对齐,因为不同的编辑器和操作系统可能会对制表符的宽度进行不同的解释。另外,也可以使用代码格式化工具对代码进行自动对齐,例如Black和YAPF等工具。
相关问题
python 制表符
在 Python 中,制表符是用来缩进代码块的特殊字符。它通常用于定义代码的结构和层次关系,例如在函数、循环或条件语句中。
制表符在 Python 中使用"\t"来表示。当你在代码中使用一个制表符,它会被解释为等同于四个空格的缩进。
例如,下面是一个使用制表符缩进的示例:
```python
def print_numbers():
for i in range(1, 5):
print("\t", i)
print_numbers()
```
在上面的例子中,我们定义了一个名为`print_numbers`的函数,并在该函数内部使用制表符缩进来打印数字1到4。运行这段代码将输出以下内容:
```
1
2
3
4
```
请注意,虽然我们在这个例子中使用了单个制表符缩进,但通常推荐使用四个空格进行缩进。这样做可以确保在不同的编辑器和环境中代码的可读性和一致性。
python制表符设置
在Python中,制表符(Tab)可以用来缩进代码块,以便更好地组织和可读性。默认情况下,Python使用四个空格作为制表符的宽度。但是,你可以根据个人偏好进行设置。
如果你想要改变制表符的宽度,可以使用`tabsize`参数来设置。例如,如果你想将制表符宽度设置为两个空格,你可以在代码中添加以下行:
```python
import sys
sys.stdout.write("\x1b[3;{0}t".format(2))
```
这将把制表符的宽度设置为两个空格。
另外,如果你使用的是文本编辑器或集成开发环境(IDE),你也可以在其设置中找到选项来更改制表符的宽度。这样,你就可以在编辑器中进行设置,而不必在代码中进行更改。
记住,在编写代码时,一致性非常重要。与其他开发者合作时,最好遵循团队的约定和项目规范。
相关推荐
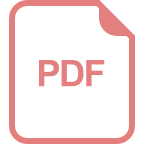
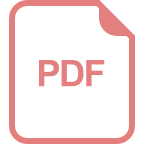
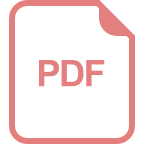












